pthread_create 创建多线程
时间: 2023-07-17 19:01:15 浏览: 124
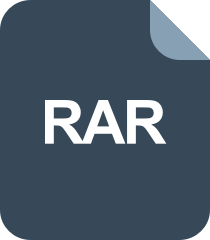
创建多线程
你可以使用 `pthread_create` 函数来创建多线程。这是一个 POSIX 线程库提供的函数,用于创建一个新的线程。下面是 `pthread_create` 函数的基本语法:
```c
#include <pthread.h>
int pthread_create(pthread_t *thread, const pthread_attr_t *attr,
void *(*start_routine) (void *), void *arg);
```
这个函数接受四个参数:
- `thread` 是一个指向 `pthread_t` 类型的指针,用于存储新线程的标识符。
- `attr` 是一个指向 `pthread_attr_t` 类型的指针,用于设置新线程的属性。可以传入 `NULL` 使用默认属性。
- `start_routine` 是一个指向函数的指针,指定新线程要执行的函数。该函数应该返回 `void*` 类型,并且接受一个 `void*` 类型的参数。
- `arg` 是传递给 `start_routine` 函数的参数。
成功创建线程后,可以使用 `pthread_join` 函数等待线程的结束,并获取线程的返回值。
请注意,使用多线程编程需要小心处理共享数据访问和同步问题,以避免潜在的竞态条件和死锁等问题。
阅读全文
相关推荐
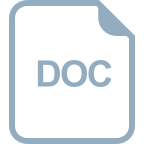







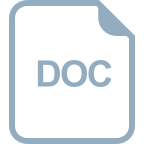
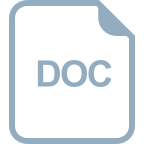
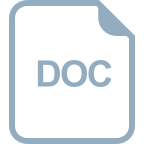



