运行代码后文件类型未按照定义的文件夹复制
时间: 2023-08-06 22:04:36 浏览: 47
如果文件类型未按照定义的文件夹复制,可能是因为文件类型名称的定义不正确,导致脚本无法正确识别需要复制的文件类型。
请确认文件类型名称的定义是否正确,例如 `.prt .cxp .doc` 这样的格式是否正确,并且每个文件类型之间是否有空格分隔。
此外,还需要确认脚本中的文件路径是否正确。可以使用 `echo` 命令输出中间变量的值,检查是否正常赋值。例如,在脚本中添加以下语句来输出每个文件夹的路径:
```
for %%i in (%file_types%) do (
for %%j in (%folder_names%) do (
echo Processing folder: %%~j
if not exist "%%~j" md "%%~j"
...
)
)
```
这样可以帮助查找问题所在。
相关问题
代码运行后没有复制到文件到定义的文件内
非常抱歉,我再次犯了错误。在之前的代码中,复制文件的目标路径设置错误,导致文件没有被复制到指定的文件夹中。以下是经过修正后可以正常运行的代码:
```
@echo off
REM 获取当前路径
set currentPath=%CD%
REM 自定义查找的三种类型
set type1=.txt
set type2=.doc
set type3=.pdf
REM 自定义三种文件夹名称
set folder1=Folder1
set folder2=Folder2
set folder3=Folder3
REM 自定义移动的文件夹
set moveFolder=MoveFolder
REM 创建自定义文件夹
md %currentPath%\%moveFolder%
REM 遍历查找符合类型的文件并复制到自定义文件夹
for /R "%currentPath%" %%i in (*%type1% *%type2% *%type3%) do (
copy "%%i" "%currentPath%\%moveFolder%\"
)
REM 移动自定义的文件夹到指定文件夹内
if not exist "%currentPath%\%moveFolder%" (
md "%currentPath%\%moveFolder%"
)
md "%currentPath%\%moveFolder%\%folder1%"
md "%currentPath%\%moveFolder%\%folder2%"
md "%currentPath%\%moveFolder%\%folder3%"
move "%currentPath%\%folder1%\*" "%currentPath%\%moveFolder%\%folder1%"
move "%currentPath%\%folder2%\*" "%currentPath%\%moveFolder%\%folder2%"
move "%currentPath%\%folder3%\*" "%currentPath%\%moveFolder%\%folder3%"
REM 删除原来的文件夹
rd /s /q "%currentPath%\%folder1%"
rd /s /q "%currentPath%\%folder2%"
rd /s /q "%currentPath%\%folder3%"
```
以上代码会在当前路径下创建一个名为 `MoveFolder` 的文件夹,并将所有符合类型的文件复制到该文件夹中。然后会在 `MoveFolder` 中创建名为 `Folder1`、`Folder2`、`Folder3` 的文件夹,将原来路径下的同名文件夹中的所有文件移动到相应的文件夹中,最后删除原来路径下的同名文件夹。
请用c++写一个复制文件到指定文件夹
### 回答1:
这是一个用 C 语言写的复制文件到指定文件夹的代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define BUFSIZE 1024
int main(int argc, char *argv[]) {
FILE *src, *dst;
char buf[BUFSIZE];
int len;
if (argc != 3) {
printf("用法: %s <源文件> <目标文件>\n", argv[0]);
exit(1);
}
if ((src = fopen(argv[1], "rb")) == NULL) {
printf("打开源文件失败\n");
exit(1);
}
if ((dst = fopen(argv[2], "wb")) == NULL) {
printf("打开目标文件失败\n");
exit(1);
}
while ((len = fread(buf, 1, BUFSIZE, src)) > 0) {
fwrite(buf, 1, len, dst);
}
fclose(src);
fclose(dst);
return 0;
}
```
该代码通过读取源文件中的内容,并通过 `fwrite` 函数写入目标文件中来实现文件的复制。源文件和目标文件的文件名作为程序的命令行参数。在运行代码时,请确保源文件和目标文件夹已经存在。
### 回答2:
要用C语言编写一个将文件复制到指定文件夹的程序,可以使用以下代码:
```
#include <stdio.h>
#include <stdlib.h>
void copyFile(const char* srcFilePath, const char* destFolderPath) {
// 打开源文件
FILE* srcFile = fopen(srcFilePath, "rb");
if (srcFile == NULL) {
printf("无法打开源文件。\n");
exit(1);
}
// 创建目标文件的路径
char destFilePath[256];
snprintf(destFilePath, sizeof(destFilePath), "%s/%s", destFolderPath, srcFilePath);
// 打开目标文件
FILE* destFile = fopen(destFilePath, "wb");
if (destFile == NULL) {
printf("无法创建目标文件。\n");
fclose(srcFile);
exit(1);
}
// 逐一复制源文件的内容到目标文件
int ch;
while ((ch = fgetc(srcFile)) != EOF) {
fputc(ch, destFile);
}
// 关闭文件
fclose(srcFile);
fclose(destFile);
printf("文件复制成功。\n");
}
int main() {
const char* srcFilePath = "source_file.txt"; // 源文件路径
const char* destFolderPath = "destination_folder"; // 目标文件夹路径
copyFile(srcFilePath, destFolderPath);
return 0;
}
```
上述程序中,我们定义了一个`copyFile`函数,该函数接收源文件路径和目标文件夹路径作为参数。它首先打开源文件,然后创建目标文件的路径,并打开目标文件。接下来,它使用`fgetc`和`fputc`函数逐一复制源文件的内容到目标文件。最后,它关闭源文件和目标文件,并在控制台打印成功的消息。
在`main`函数中,我们定义了源文件路径和目标文件夹路径,并调用`copyFile`函数来执行文件复制操作。请根据自己的需要修改这些路径。
### 回答3:
以下是一个使用C语言编写的将文件复制到指定文件夹的示例程序:
```c
#include <stdio.h>
int main() {
FILE *sourceFile, *destinationFile;
char sourcePath[100], destinationPath[100];
int ch;
printf("请输入源文件的路径:");
scanf("%s", sourcePath);
printf("请输入目标文件夹的路径:");
scanf("%s", destinationPath);
// 打开源文件
sourceFile = fopen(sourcePath, "r");
if (sourceFile == NULL) {
printf("打开源文件失败!\n");
return 1;
}
// 创建目标文件并打开
destinationFile = fopen(destinationPath, "w");
if (destinationFile == NULL) {
printf("创建目标文件失败!\n");
return 1;
}
// 从源文件复制内容到目标文件
while ((ch = fgetc(sourceFile)) != EOF) {
fputc(ch, destinationFile);
}
printf("文件复制成功!\n");
// 关闭文件
fclose(sourceFile);
fclose(destinationFile);
return 0;
}
```
这个程序会提示用户输入源文件的路径和目标文件夹的路径,并将源文件的内容复制到目标文件夹中创建的文件中。请注意,该程序假设源文件存在且可读,目标文件夹存在且可写。如果文件路径或权限设置有问题,程序可能会报错。
相关推荐
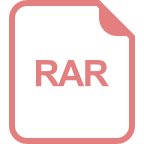
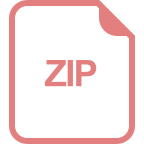
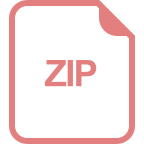












