举一个使用python os库把一个文件夹中的所有文件复制到另一个文件夹
时间: 2023-08-31 08:38:53 浏览: 94
### 回答1:
可以使用以下代码实现:
import os
import shutil
# 原文件夹路径
src_folder = "/path/to/source/folder"
# 目标文件夹路径
dst_folder = "/path/to/destination/folder"
# 获取原文件夹中的所有文件
files = os.listdir(src_folder)
# 遍历所有文件并复制到目标文件夹中
for file in files:
src_file = os.path.join(src_folder, file)
dst_file = os.path.join(dst_folder, file)
shutil.copy(src_file, dst_file)
注意,需要先导入os和shutil库,然后指定原文件夹路径和目标文件夹路径,使用os.listdir()函数获取原文件夹中的所有文件,然后遍历所有文件,使用shutil.copy()函数将文件复制到目标文件夹中。
### 回答2:
使用Python的os库来复制一个文件夹中的所有文件到另一个文件夹的方法如下:
首先,我们需要导入os库。
```python
import os
```
接下来,定义一个函数来完成文件夹内文件复制的功能。
```python
def copy_files(source_folder, destination_folder):
for root, dirs, files in os.walk(source_folder):
for file in files:
source_path = os.path.join(root, file) # 源文件的完整路径
destination_path = os.path.join(destination_folder, file) # 目标文件的完整路径
os.makedirs(os.path.dirname(destination_path), exist_ok=True) # 创建目标文件夹
try:
with open(source_path, 'rb') as source_file, open(destination_path, 'wb') as destination_file:
destination_file.write(source_file.read()) # 复制文件内容
print(f"成功复制文件 {file}")
except IOError:
print(f"无法复制文件 {file}")
```
在这个函数中,我们使用``os.walk(source_folder)``来递归遍历源文件夹中的所有文件和子文件夹。然后,我们通过``os.path.join()``函数拼接文件的完整路径。
在进行复制之前,我们使用``os.makedirs()``函数创建目标文件夹,如果目标文件夹已经存在,则不进行创建。
然后,我们使用``with open(source_path, 'rb')``来打开源文件,并使用``with open(destination_path, 'wb')``来创建目标文件。接着,我们使用``source_file.read()``读取源文件内容,并使用``destination_file.write()``将内容写入目标文件中,实现文件的复制。
最后,我们使用一个try-except语句来处理复制过程中可能出现的IOError异常,并在复制成功或失败时打印相应的消息。
接下来,我们可以调用这个函数来复制文件夹中的文件。
```python
source_folder = '源文件夹路径'
destination_folder = '目标文件夹路径'
copy_files(source_folder, destination_folder)
```
请确保将`源文件夹路径`和`目标文件夹路径`替换为实际的文件夹路径。运行程序后,它将递归地复制源文件夹中的所有文件和子文件夹到目标文件夹中,并在完成每个复制操作后打印相应的消息。
这是使用Python的os库将一个文件夹中的所有文件复制到另一个文件夹的简单方法。
### 回答3:
使用Python的os库可以很方便地实现将一个文件夹中的所有文件复制到另一个文件夹。具体步骤如下:
1. 首先导入os库:
```python
import os
```
2. 定义函数以实现复制文件:
```python
def copy_files(src, dst):
for file_name in os.listdir(src): # 遍历源文件夹中的所有文件
src_file = os.path.join(src, file_name) # 源文件的完整路径
dst_file = os.path.join(dst, file_name) # 目标文件的完整路径
if os.path.isfile(src_file): # 判断是否为文件
with open(src_file, 'rb') as fsrc:
with open(dst_file, 'wb') as fdst:
fdst.write(fsrc.read()) # 将源文件内容复制到目标文件
elif os.path.isdir(src_file): # 判断是否为文件夹
os.makedirs(dst_file, exist_ok=True) # 创建目标文件夹
copy_files(src_file, dst_file) # 递归调用函数复制文件夹中的文件
```
3. 调用函数复制文件夹中的所有文件:
```python
src_folder = "源文件夹路径"
dst_folder = "目标文件夹路径"
copy_files(src_folder, dst_folder)
```
以上代码会将源文件夹中的所有文件复制到目标文件夹中。如果目标文件夹不存在,则会自动创建。同时,如果源文件夹中包含子文件夹,也会一并复制其中的文件到目标文件夹中。
相关推荐
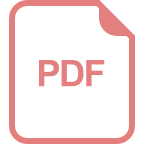
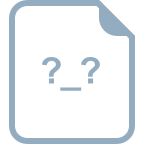














