python将一个文件夹中的所有文件复制到另一个文件夹的代码
时间: 2024-05-10 21:16:54 浏览: 186
可以使用Python的shutil模块来实现文件复制功能。
下面是一个将源目录(source_dir)中的所有文件复制到目标目录(target_dir)的Python代码示例:
```python
import os
import shutil
# 源目录
source_dir = '/path/to/source/folder'
# 目标目录
target_dir = '/path/to/target/folder'
# 遍历源目录中的所有文件
for file_name in os.listdir(source_dir):
# 源文件的完整路径
src_file = os.path.join(source_dir, file_name)
# 判断源文件是否是文件夹
if os.path.isdir(src_file):
continue
# 目标文件的完整路径
dst_file = os.path.join(target_dir, file_name)
# 复制文件
shutil.copy(src_file, dst_file)
```
在上面的代码中,首先定义了源目录和目标目录的路径。然后使用os.listdir()函数遍历源目录中的所有文件,判断每个文件是否是文件夹,如果是文件夹则跳过。使用os.path.join()函数拼接源文件的完整路径和目标文件的完整路径,然后使用shutil.copy()函数复制文件。
相关问题
使用Python将一个文件夹下的多个文件夹中的文件复制到一个文件夹中
以下是使用Python将一个文件夹下的多个文件夹中的文件复制到一个文件夹中的示例代码:
```python
import os
import shutil
source_path = "/path/to/source/folder"
target_path = "/path/to/target/folder"
# 遍历源文件夹下所有文件夹
for subdir, dirs, files in os.walk(source_path):
# 遍历当前文件夹下所有文件
for file in files:
# 构建源文件路径和目标文件路径
source_file = os.path.join(subdir, file)
target_file = os.path.join(target_path, file)
# 复制文件到目标文件夹
shutil.copy2(source_file, target_file)
```
在上面的代码中,我们首先定义了源文件夹和目标文件夹的路径,然后使用os.walk函数遍历源文件夹下的所有文件夹和文件。对于每个文件,我们使用os.path.join函数构建源文件路径和目标文件路径,然后使用shutil.copy2函数将文件复制到目标文件夹中。最终,所有文件都将被复制到目标文件夹中。
python实现将文件夹中的xlsx文件复制到另一个文件夹中,其他文件不复制
您可以使用Python的shutil模块来实现将文件夹中的xlsx文件复制到另一个文件夹中的功能。以下是一个示例代码:
```python
import os
import shutil
def copy_xlsx_files(source_folder, destination_folder):
# 检查目标文件夹是否存在,如果不存在则创建
if not os.path.exists(destination_folder):
os.makedirs(destination_folder)
# 遍历源文件夹中的文件
for filename in os.listdir(source_folder):
source_file = os.path.join(source_folder, filename)
# 检查文件是否为xlsx文件
if filename.endswith('.xlsx') and os.path.isfile(source_file):
destination_file = os.path.join(destination_folder, filename)
shutil.copy2(source_file, destination_file)
print("xlsx文件复制完成!")
# 调用函数进行复制
copy_xlsx_files("源文件夹路径", "目标文件夹路径")
```
请将"源文件夹路径"和"目标文件夹路径"替换为您实际的文件夹路径。这段代码将遍历源文件夹中的所有文件,如果文件是以".xlsx"结尾的xlsx文件,则将其复制到目标文件夹中。
阅读全文
相关推荐
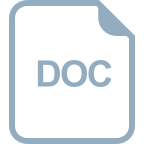














