优化以下代码,要求:班级成绩管理系统 (1)利用结构体和简单的链表(关于链表的知识,自己查阅资料学习),实现一个班学生成绩的管理。 (2)成绩管理功能包括:输入、输出,利用new和delete运算符添加/删除班级成绩管理系统 (1)利用结构体和简单的链表(关于链表的知识,自己查阅资料学习),实现一个班学生成绩的管理。 (2)成绩管理功能包括:输入、输出,利用new和delete运算符添加/删除学生信息。#include<stdio.h> #include<iostream> #include<windows.h> using namespace std; typedef struct student { char name[20]; int score; student* next; }stu; stu* head; void input() { stu* p1, * p2; p1 = (stu*)new stu; head = p1; while (p1 != NULL) { cout << "输入姓名" << endl; cin >> p1->name; cout << "输入成绩" << endl; cin >> p1->score; cout << "是否继续输入?(1.是 2.否)"; int a; cin >> a; if (a == 1) { p2 = p1; p1 = (stu*)new stu; p2->next = p1; continue; } else if (a == 2) { p1->next = NULL; break; } } } void listprint() { stu* p1 = head; while (p1 != NULL) { cout << 1; cout << "姓名: " << p1->name << " 成绩:" << p1->score << endl; p1 = p1->next; } } void shanchu() { char c[20]; cout << "请输入删除的学生姓名" << endl; cin >> c; stu* p1 = head, * p2; while (p1 != NULL) { p2 = p1->next; if (strcmp(p2->name, c) == 0) { p1->next = p2->next; break; }p1 = p1->next; } } int main() { int a = 0; while (1) { cout << "1.输入成绩 2.输出成绩 3.删除" << endl;cin >> a; switch (a) { case 1: { input(); system ("cls"); continue; } case 2: { listprint(); continue; } case 3: { shanchu(); continue; } default: { break; } } break; } }
时间: 2023-04-07 20:01:08 浏览: 112
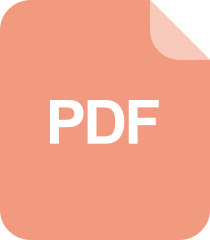
C语言利用结构体数组实现学生成绩管理系统

以下是优化后的代码:
#include <iostream>
#include <string>
using namespace std;
struct Student {
string name;
int score;
Student* next;
};
void addStudent(Student*& head, string name, int score) {
Student* newStudent = new Student;
newStudent->name = name;
newStudent->score = score;
newStudent->next = head;
head = newStudent;
}
void deleteStudent(Student*& head, string name) {
if (head == NULL) {
return;
}
if (head->name == name) {
Student* temp = head;
head = head->next;
delete temp;
return;
}
Student* current = head;
while (current->next != NULL && current->next->name != name) {
current = current->next;
}
if (current->next == NULL) {
return;
}
Student* temp = current->next;
current->next = current->next->next;
delete temp;
}
void printStudents(Student* head) {
while (head != NULL) {
cout << head->name << " " << head->score << endl;
head = head->next;
}
}
int main() {
Student* head = NULL;
addStudent(head, "Alice", 90);
addStudent(head, "Bob", 80);
addStudent(head, "Charlie", 70);
printStudents(head);
deleteStudent(head, "Bob");
printStudents(head);
return 0;
}
这个代码实现了一个班级成绩管理系统,使用了结构体和简单的链表。其中,结构体Student表示一个学生,包括姓名和成绩,以及一个指向下一个学生的指针。函数addStudent用于添加一个学生,将其插入到链表的头部;函数deleteStudent用于删除一个学生,根据姓名查找并删除;函数printStudents用于输出所有学生的姓名和成绩。在main函数中,我们先添加了三个学生,然后输出所有学生的信息,接着删除了一个学生,再次输出所有学生的信息。
阅读全文
相关推荐
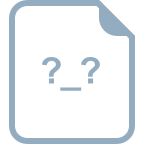
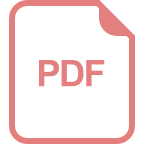

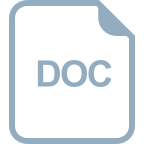
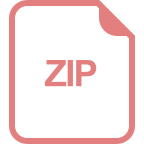
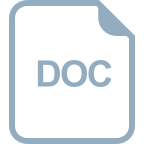
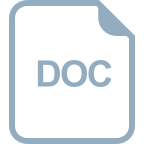
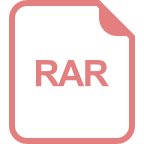
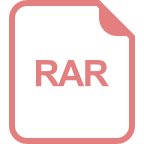
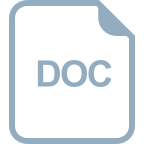
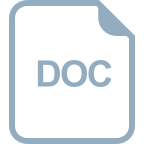

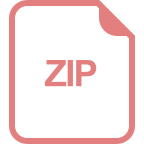