stm32f407 标准库 编写 adc 定时中断 GPIO的个人库函数
时间: 2024-08-17 16:01:49 浏览: 58
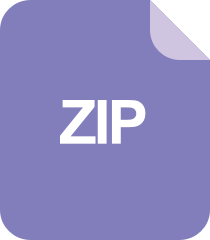
STM32F407库函数模板
STM32F407系列微控制器的标准库中并没有直接提供ADC定时中断和GPIO操作的个人库函数。然而,你可以通过标准库函数如`HAL_ADC_Start`, `HAL_ADC_IRQHandler`, 和`HAL_GPIO_Init`等来进行这些功能的自定义整合。
首先,你需要初始化ADC模块,例如:
```c
void init_adc(void) {
ADC_HandleTypeDef hadc1; // 创建ADC handle
hadc1.Instance = ADC1; // 设置ADC实例
HAL_ADC_Init(&hadc1); // 初始化ADC配置
// 配置定时器用于触发ADC转换
TIM_HandleTypeDef htim1;
... (配置TIM1为ADC外部触发)
HAL_TIM_Base_Start(&htim1);
}
```
然后,设置ADC的中断处理函数,当转换完成时会被调用:
```c
void MX_ADC1_IRQHandler(void) {
HAL_ADC_IRQHandler(&hadc1); // 处理ADC中断
if (hadc1.State == HAL_ADC_STATE_COMPLETED) {
process_adc_data(); // 处理获取到的数据
}
}
```
对于GPIO的操作,比如配置输入或输出,可以这样做:
```c
void setup_gpio(uint8_t gpio_pin, PinState state) {
GPIO_InitTypeDef GPIO_InitStruct;
GPIO_InitStruct.Pin = GPIO_PIN_x; // replace x with your GPIO pin number
GPIO_InitStruct.Mode = GPIO_MODE_INPUT_OUTPUT; // 输入输出模式
GPIO_InitStruct.Pull = state ? GPIO_NOPULL : GPIO_PULLDOWN; // 根据需要设置拉高或拉低
GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH;
HAL_GPIO_Init(GPIOx, &GPIO_InitStruct); // GPIOx is the GPIO port register, like GPIOA for example
}
```
记得在适当的时候注册ADC的中断,并开启它:
```c
void enable_adc_timer_and_int(void) {
// Enable ADC interrupt and set callback function
__HAL_ADC_ENABLE_IT(&hadc1, ADC_IT_EOC);
// Register ADC1 interruption handler
HAL_NVIC_SetPriority(ADC1_IRQn, YOUR_INTERRUPT_PRIORITY, 0);
HAL_NVIC_EnableIRQ(ADC1_IRQn);
}
```
阅读全文
相关推荐
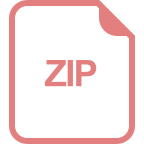
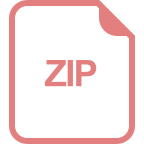


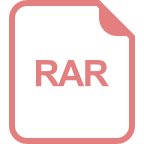
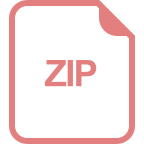
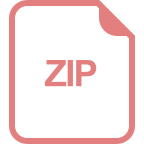
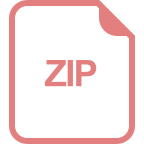
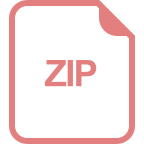
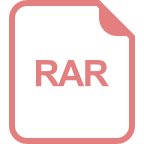
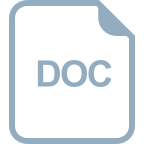
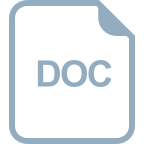
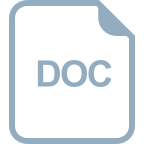




