1.编程实现一个学生对象,要求有姓名、性别、年龄,输出对应的姓名、性别和年龄。 2.编程实现:定义一个动物Animal类,类中有属性名字和年龄和吃的方法,再定义一个狗(Dog)和猫 (Cat)的类,分别继承动物类,输出动物的姓名和年龄并发出不同的叫声。
时间: 2024-10-27 15:13:56 浏览: 40
当然,让我们分步骤来创建这些类和对象。
1. 首先,我们可以创建一个简单的`Student`对象,包含姓名、性别和年龄:
```javascript
class Student {
constructor(name, gender, age) {
this.name = name;
this.gender = gender;
this.age = age;
}
// 输出学生信息
displayInfo() {
console.log(`Name: ${this.name}`);
console.log(`Gender: ${this.gender}`);
console.log(`Age: ${this.age}`);
}
}
// 创建一个实例并显示信息
let student = new Student('John Doe', 'Male', 20);
student.displayInfo();
```
2. 接下来,我们创建一个`Animal`基类和它的子类`Dog`和`Cat`:
```javascript
class Animal {
constructor(name, age) {
this.name = name;
this.age = age;
}
// 定义通用的吃的行为
eat() {
console.log(`${this.name} is eating.`);
}
displayInfo() {
console.log(`Name: ${this.name}`);
console.log(`Age: ${this.age}`);
}
}
class Dog extends Animal {
bark() {
console.log(`${this.name} says woof!`);
}
}
class Cat extends Animal {
meow() {
console.log(`${this.name} says meow!`);
}
}
// 创建Dog和Cat实例并展示信息
let dog = new Dog('Rex', 5);
dog.eat(); // Rex is eating.
dog.bark();
let cat = new Cat('Misty', 3);
cat.eat(); // Misty is eating.
cat.meow();
```
现在,你可以运行这段代码,你会看到学生的信息以及狗和猫的姓名、年龄和叫声。
阅读全文
相关推荐
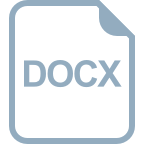
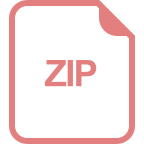


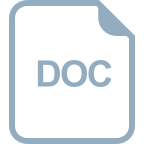
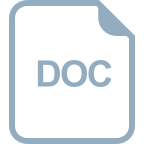
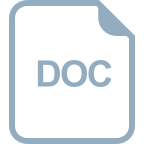
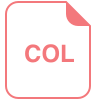
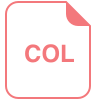
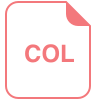
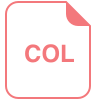





