如何用C语言编写程序来计算不同学生选修课程的成绩GPA?
时间: 2024-10-22 18:12:02 浏览: 26
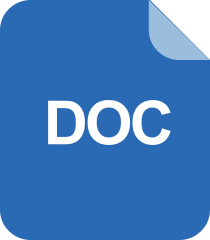
用c语言编写学生成绩管理系统.doc

在C语言中,计算学生的GPA通常涉及到以下几个步骤:
1. 定义结构体(struct)来表示学生信息,包含字段如学号(student_id)、姓名(name)、以及每个课程的成绩(scores)和对应的学分(credit_hours)。例如:
```c
typedef struct {
int student_id;
char name[50];
float scores[10]; // 假设最多选修10门课程
int credit_hours[10];
} Student;
```
2. 创建函数来计算单个课程的绩点(GPA_per_course),这通常通过将分数除以学分转换而来,并根据常见的绩点系统(比如4.0制)进行调整。
3. 遍历学生的所有成绩,计算总绩点(total_gpa)。这个过程需要遍历scores数组并累加每个课程的绩点乘以其相应的学分。
4. 最后,用总绩点除以总学分得到平均GPA(average_gpa)。如果某个学生未选修任何课程,则考虑是否允许0学分GPA或者报错处理。
这是一个基本示例:
```c
// 计算单科绩点
float calculate_gpa(float score, int credit_hours) {
return score / credit_hours;
}
// 主函数
int main() {
Student students[] = {
{1, "张三", {85, 90, 88}, {3, 4, 2}},
// 其他学生...
};
for (int i = 0; i < sizeof(students)/sizeof(students[0]); i++) {
float total_gpa = 0;
int total_credit_hours = 0;
for (int j = 0; j < 3; j++) { // 假设每个学生最多有3门课
total_gpa += calculate_gpa(students[i].scores[j], students[i].credit_hours[j]);
total_credit_hours += students[i].credit_hours[j];
}
if (total_credit_hours > 0) {
float average_gpa = total_gpa / total_credit_hours;
printf("学生 %d 的 GPA: %.2f\n", students[i].student_id, average_gpa);
} else {
printf("学生 %d 没有选修任何课程\n", students[i].student_id);
}
}
return 0;
}
```
阅读全文
相关推荐
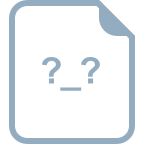
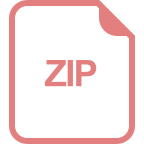
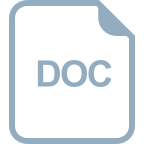
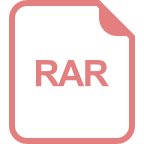
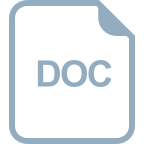
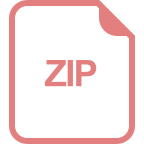
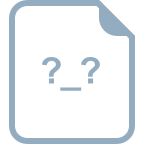
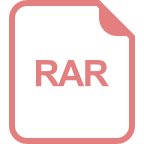
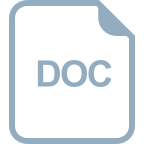
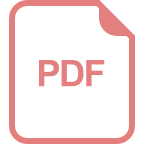
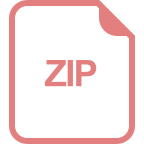
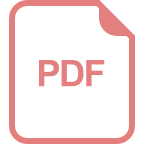