使用JSP+Servlet+JDBC技术 三层架构 对atm库中的atm_user表进行 1,登录 2,列表(user_name条件模糊查询) 3,删除
时间: 2023-11-22 19:56:44 浏览: 53
好的,我可以为您提供一个简单的示例代码。
首先,我们需要建立一个三层架构的项目,包含 DAO 层、Service 层和 Controller 层。
在 DAO 层中,我们可以定义一个 UserDao 接口和一个 UserDaoImpl 类来实现该接口。在 UserDao 接口中,我们可以定义登录、列表和删除等操作的方法。在 UserDaoImpl 类中,我们可以使用 JDBC 技术来实现这些方法。
在 Service 层中,我们可以定义一个 UserService 接口和一个 UserServiceImpl 类来实现该接口。在 UserServiceImpl 类中,我们可以调用 UserDaoImpl 中的方法,并对数据进行一些处理和封装。
在 Controller 层中,我们可以定义一个 UserServlet 类来处理用户请求。在 UserServlet 类中,我们可以调用 UserServiceImpl 中的方法,并将结果发送给用户界面。
下面是示例代码:
UserDao.java
```java
public interface UserDao {
boolean login(String username, String password) throws SQLException;
List<User> getUserList(String username) throws SQLException;
boolean deleteUser(int id) throws SQLException;
}
```
UserDaoImpl.java
```java
public class UserDaoImpl implements UserDao {
private Connection conn = null;
private PreparedStatement pstmt = null;
public UserDaoImpl(Connection conn) {
this.conn = conn;
}
@Override
public boolean login(String username, String password) throws SQLException {
String sql = "SELECT * FROM atm_user WHERE user_name=? AND user_password=?";
pstmt = conn.prepareStatement(sql);
pstmt.setString(1, username);
pstmt.setString(2, password);
ResultSet rs = pstmt.executeQuery();
if (rs.next()) {
return true;
} else {
return false;
}
}
@Override
public List<User> getUserList(String username) throws SQLException {
List<User> userList = new ArrayList<>();
String sql = "SELECT * FROM atm_user WHERE user_name LIKE ?";
pstmt = conn.prepareStatement(sql);
pstmt.setString(1, "%" + username + "%");
ResultSet rs = pstmt.executeQuery();
while (rs.next()) {
User user = new User();
user.setId(rs.getInt("user_id"));
user.setUsername(rs.getString("user_name"));
user.setPassword(rs.getString("user_password"));
user.setBalance(rs.getDouble("user_balance"));
userList.add(user);
}
return userList;
}
@Override
public boolean deleteUser(int id) throws SQLException {
String sql = "DELETE FROM atm_user WHERE user_id=?";
pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, id);
int result = pstmt.executeUpdate();
if (result > 0) {
return true;
} else {
return false;
}
}
}
```
UserService.java
```java
public interface UserService {
boolean login(String username, String password) throws SQLException;
List<User> getUserList(String username) throws SQLException;
boolean deleteUser(int id) throws SQLException;
}
```
UserServiceImpl.java
```java
public class UserServiceImpl implements UserService {
private UserDao userDao;
public UserServiceImpl(UserDao userDao) {
this.userDao = userDao;
}
@Override
public boolean login(String username, String password) throws SQLException {
return userDao.login(username, password);
}
@Override
public List<User> getUserList(String username) throws SQLException {
return userDao.getUserList(username);
}
@Override
public boolean deleteUser(int id) throws SQLException {
return userDao.deleteUser(id);
}
}
```
UserServlet.java
```java
public class UserServlet extends HttpServlet {
private UserService userService;
public UserServlet() {
super();
userDao = new UserDaoImpl(DBUtil.getConnection());
userService = new UserServiceImpl(userDao);
}
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doPost(request, response);
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String action = request.getParameter("action");
if (action.equals("login")) {
String username = request.getParameter("username");
String password = request.getParameter("password");
try {
if (userService.login(username, password)) {
response.sendRedirect("userList.jsp");
} else {
response.sendRedirect("login.jsp");
}
} catch (SQLException e) {
e.printStackTrace();
}
} else if (action.equals("list")) {
String username = request.getParameter("username");
try {
List<User> userList = userService.getUserList(username);
request.setAttribute("userList", userList);
RequestDispatcher rd = request.getRequestDispatcher("userList.jsp");
rd.forward(request, response);
} catch (SQLException e) {
e.printStackTrace();
}
} else if (action.equals("delete")) {
int id = Integer.parseInt(request.getParameter("id"));
try {
userService.deleteUser(id);
response.sendRedirect("userList.jsp");
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
```
以上代码仅供参考,具体的实现还需要您根据自己的需求进行修改。希望对您有所帮助!
相关推荐
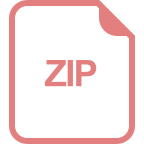
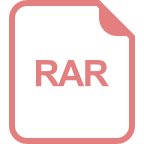














