vue中iframe嵌套页面传值
时间: 2023-09-24 16:13:53 浏览: 152
在Vue中,如果需要在iframe嵌套页面之间传值,可以使用window.postMessage()方法。
例如,父页面中有一个iframe:
```html
<iframe src="https://example.com"></iframe>
```
在父页面中定义一个方法,用于向iframe发送数据:
```javascript
function sendMessage(data) {
const iframe = document.querySelector('iframe');
iframe.contentWindow.postMessage(data, 'https://example.com');
}
```
在iframe中定义一个事件监听器,用于接收来自父页面的数据:
```javascript
window.addEventListener('message', (event) => {
if (event.origin !== 'https://example.com') return;
const data = event.data;
// 处理接收到的数据
});
```
在接收到数据后,可以在iframe中进行相应的处理。注意要判断消息来源的origin,确保数据来自正确的页面。
相关问题
vue中iframe嵌套页面并传值
在Vue中,可以通过给iframe的src属性传递参数的方式来实现iframe嵌套页面传值。
1. 在父组件中,定义一个变量来存储要传递给iframe的参数值。
```javascript
<template>
<div>
<iframe :src="iframeUrl"></iframe>
</div>
</template>
<script>
export default {
data() {
return {
iframeUrl: ''
}
},
mounted() {
// 设置iframe的src属性,传递参数
this.iframeUrl = `/iframe?param=${this.paramValue}`;
},
computed: {
paramValue() {
// 从父组件中获取参数值
return this.$store.state.paramValue;
}
}
}
</script>
```
2. 在iframe的嵌套页面中,通过获取url中的参数来获取父组件传递的参数值。
```javascript
<template>
<div>{{ param }}</div>
</template>
<script>
export default {
data() {
return {
param: ''
}
},
mounted() {
// 获取url中的参数值
const searchParams = new URLSearchParams(window.location.search);
this.param = searchParams.get('param');
}
}
</script>
```
这样就可以实现在Vue中通过iframe嵌套页面并传值的功能。
vue中iframe嵌套页面跳转
在Vue中嵌套iframe页面跳转,可以使用iframe的window对象的location属性进行页面跳转。
例如,在Vue组件中嵌套一个iframe:
```
<template>
<div>
<iframe ref="myIframe" src="http://www.example.com"></iframe>
</div>
</template>
```
然后,在Vue组件的方法中使用iframe的window对象的location属性进行页面跳转:
```
<script>
export default {
methods: {
goToPage() {
this.$refs.myIframe.contentWindow.location.href = 'http://www.example.com/newpage';
}
}
}
</script>
```
在这个例子中,当调用goToPage方法时,会将iframe中的页面跳转到http://www.example.com/newpage。
阅读全文
相关推荐
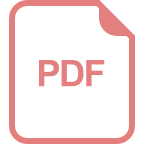
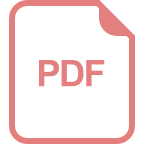

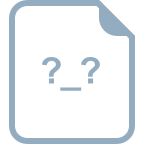
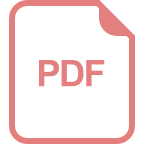
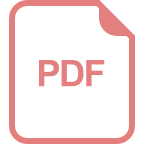
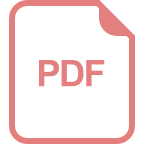
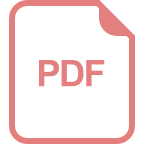
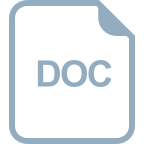





