请用c语言编写linux环境下运行的一个程序,其功能为实现生产者消费者功能。缓冲区大小为10,有3个生产者进程,4个消费者进程。要求生产者任务从文件中读取数据,并将它存放到缓冲区,而消费者任务从缓冲区中取走此数据,并打印输出。
时间: 2024-02-22 20:55:18 浏览: 27
好的,这是一个经典的生产者消费者问题。我给你提供一个基本的代码框架,你可以在此基础上进行修改和完善。
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define BUFFER_SIZE 10
#define PRODUCER_NUM 3
#define CONSUMER_NUM 4
int buffer[BUFFER_SIZE];
int in = 0;
int out = 0;
int count = 0;
pthread_mutex_t mutex;
pthread_cond_t full;
pthread_cond_t empty;
void *producer(void *arg)
{
FILE *fp;
char line[100];
fp = fopen("data.txt", "r");
if (fp == NULL) {
perror("Error opening file");
exit(EXIT_FAILURE);
}
while (fgets(line, sizeof(line), fp)) {
int item = atoi(line);
pthread_mutex_lock(&mutex);
while (count == BUFFER_SIZE) {
pthread_cond_wait(&full, &mutex);
}
buffer[in] = item;
in = (in + 1) % BUFFER_SIZE;
count++;
printf("Producer %ld produced %d\n", (long)arg, item);
pthread_cond_signal(&empty);
pthread_mutex_unlock(&mutex);
}
fclose(fp);
pthread_exit(NULL);
}
void *consumer(void *arg)
{
while (1) {
pthread_mutex_lock(&mutex);
while (count == 0) {
pthread_cond_wait(&empty, &mutex);
}
int item = buffer[out];
out = (out + 1) % BUFFER_SIZE;
count--;
printf("Consumer %ld consumed %d\n", (long)arg, item);
pthread_cond_signal(&full);
pthread_mutex_unlock(&mutex);
}
pthread_exit(NULL);
}
int main(int argc, char *argv[])
{
pthread_t producer_thread[PRODUCER_NUM];
pthread_t consumer_thread[CONSUMER_NUM];
int i;
pthread_mutex_init(&mutex, NULL);
pthread_cond_init(&full, NULL);
pthread_cond_init(&empty, NULL);
for (i = 0; i < PRODUCER_NUM; i++) {
pthread_create(&producer_thread[i], NULL, producer, (void *)(long)i);
}
for (i = 0; i < CONSUMER_NUM; i++) {
pthread_create(&consumer_thread[i], NULL, consumer, (void *)(long)i);
}
for (i = 0; i < PRODUCER_NUM; i++) {
pthread_join(producer_thread[i], NULL);
}
for (i = 0; i < CONSUMER_NUM; i++) {
pthread_join(consumer_thread[i], NULL);
}
pthread_mutex_destroy(&mutex);
pthread_cond_destroy(&full);
pthread_cond_destroy(&empty);
return 0;
}
```
在这个代码中,我们使用了 pthread 库来创建生产者和消费者线程,并使用了互斥锁和条件变量来实现线程之间的同步和互斥。具体来说,我们使用了一个循环缓冲区来存放生产者生产的数据,当缓冲区满时,生产者线程会阻塞等待,直到有消费者线程取走数据并唤醒生产者线程。同样地,当缓冲区为空时,消费者线程会阻塞等待,直到有生产者线程存放数据并唤醒消费者线程。在实际应用中,我们可能需要根据具体情况来调整缓冲区大小和线程数量等参数,以满足实际需求。
相关推荐
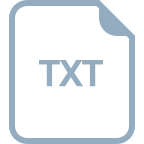
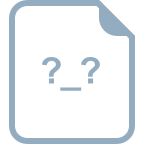
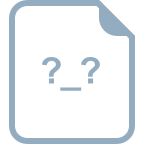














