Linux生产者消费者问题代码c语言
时间: 2024-10-24 13:01:43 浏览: 45
Linux生产者消费者问题是一个经典的并发编程示例,它涉及到两个线程角色:生产者(Producer)负责创建数据并放入缓冲区,消费者(Consumer)负责从缓冲区取出数据进行处理。这个场景通常通过互斥锁(mutex)、条件变量(condition variable)以及信号量(semaphore)来协调。
在C语言中,你可以使用`pthread.h`库来编写这样的程序。下面是一个简单的代码框架:
```c
#include <stdio.h>
#include <pthread.h>
#include <stdlib.h>
#include <semaphore.h>
#define BUFFER_SIZE 5
sem_t mutex, empty, full;
int buffer[BUFFER_SIZE] = {0};
int count = 0;
void* producer(void* arg) {
int data;
while (1) {
sem_wait(&mutex); // 获取互斥锁
if (count == BUFFER_SIZE) {
sem_wait(&full); // 等待缓冲区满
}
data = rand(); // 生产数据
buffer[count++] = data;
printf("Produced %d\n", data);
sem_post(&empty); // 通知消费者缓冲区有空位
sem_post(&mutex); // 释放互斥锁
}
return NULL;
}
void* consumer(void* arg) {
while (1) {
sem_wait(&mutex); // 获取互斥锁
if (count == 0) {
sem_wait(&empty); // 等待缓冲区有数据
}
int data = buffer[--count]; // 消费数据
printf("Consumed %d\n", data);
sem_post(&full); // 通知生产者缓冲区不满
sem_post(&mutex); // 释放互斥锁
}
return NULL;
}
int main() {
pthread_t prod_thread, cons_thread;
sem_init(&mutex, 0, 1); // 初始化互斥锁为独占状态
sem_init(&empty, 0, BUFFER_SIZE); // 初始化空信号量
sem_init(&full, 0, 0); // 初始化满信号量
if (pthread_create(&prod_thread, NULL, producer, NULL) != 0) {
perror("Producer thread creation failed");
return 1;
}
if (pthread_create(&cons_thread, NULL, consumer, NULL) != 0) {
perror("Consumer thread creation failed");
return 1;
}
pthread_join(prod_thread, NULL);
pthread_join(cons_thread, NULL);
sem_destroy(&mutex);
sem_destroy(&empty);
sem_destroy(&full);
return 0;
}
```
阅读全文
相关推荐




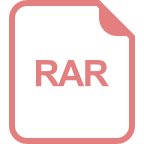

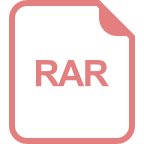











