linux用c解决生产者消费者之间的问题代码
时间: 2024-09-28 10:17:13 浏览: 45
在Linux环境下,使用C语言解决生产者消费者问题(Producer-Consumer Problem),通常会利用互斥锁(mutex)、条件变量(condition variable)或者其他同步机制来管理资源。这里提供一个简化版的示例代码:
```c
#include <pthread.h>
#include <stdio.h>
#include <stdlib.h>
#define BUFFER_SIZE 5
#define NUM_PRODUCERS 2
#define NUM_CONSUMERS 3
typedef struct {
int buffer[BUFFER_SIZE];
int count;
pthread_mutex_t mutex; // 互斥锁
pthread_cond_t empty; // 空闲信号
pthread_cond_t full; // 满载信号
} producer_consumer;
void *producer(void *arg) {
producer_consumer *pc = (producer_consumer *) arg;
while (1) {
pthread_mutex_lock(&pc->mutex);
if (pc->count == BUFFER_SIZE) {
printf("Producer %d waiting for buffer to be not full...\n", *(int*)arg);
pthread_cond_wait(&pc->full, &pc->mutex);
} else {
pc->buffer[pc->count] = *(int*)arg;
++pc->count;
printf("Producer %d produced item %d and count is now %d\n", *(int*)arg, *(int*)arg, pc->count);
pthread_cond_signal(&pc->empty); // 唤醒消费者
}
pthread_mutex_unlock(&pc->mutex);
}
}
void *consumer(void *arg) {
producer_consumer *pc = (producer_consumer *) arg;
while (1) {
pthread_mutex_lock(&pc->mutex);
if (pc->count == 0) {
printf("Consumer %d waiting for buffer to be not empty...\n", *(int*)arg);
pthread_cond_wait(&pc->empty, &pc->mutex);
} else {
int item = pc->buffer[pc->count - 1]; // 获取最后一个元素
pc->buffer[pc->count - 1] = 0; // 清空元素
--pc->count;
printf("Consumer %d consumed item %d and count is now %d\n", *(int*)arg, item, pc->count);
pthread_cond_signal(&pc->full); // 唤醒生产者
}
pthread_mutex_unlock(&pc->mutex);
}
}
int main() {
producer_consumer pc;
int i;
pthread_t producers[NUM_PRODUCERS], consumers[NUM_CONSUMERS];
pthread_mutex_init(&pc.mutex, NULL);
pthread_cond_init(&pc.empty, NULL);
pthread_cond_init(&pc.full, NULL);
for (i = 0; i < NUM_PRODUCERS; i++) {
pthread_create(&producers[i], NULL, producer, (void*)&i);
}
for (i = 0; i < NUM_CONSUMERS; i++) {
pthread_create(&consumers[i], NULL, consumer, (void*)&i);
}
for (i = 0; i < NUM_PRODUCERS; i++) {
pthread_join(producers[i], NULL);
}
for (i = 0; i < NUM_CONSUMERS; i++) {
pthread_join(consumers[i], NULL);
}
pthread_mutex_destroy(&pc.mutex);
pthread_cond_destroy(&pc.empty);
pthread_cond_destroy(&pc.full);
return 0;
}
```
这个代码示例创建了两个生产者线程和三个消费者线程,通过互斥锁和条件变量控制生产者填满缓冲区和消费者消费缓冲区的过程。每个线程都有一个循环,在满足条件后继续生产或消费。
阅读全文
相关推荐
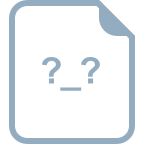
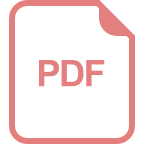
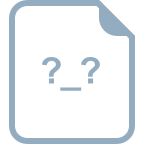
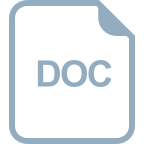



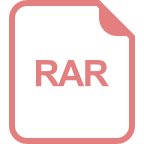
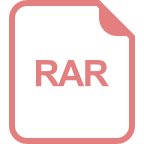
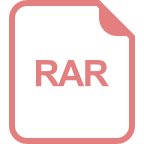
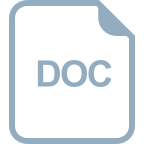
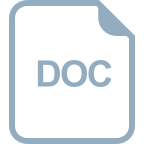
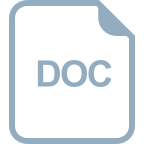
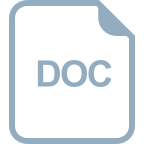




