Linux生产者消费者问题代码
时间: 2024-01-21 14:41:21 浏览: 25
下面是一个简单的 Linux 生产者消费者问题的代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#include <semaphore.h>
#define BUFFER_SIZE 10
int buffer[BUFFER_SIZE];
int in = 0;
int out = 0;
sem_t mutex, empty, full;
void *producer(void *arg) {
int i;
for (i = 0; i < 20; i++) {
sem_wait(&empty);
sem_wait(&mutex);
buffer[in] = i;
printf("Producer produced item %d at index %d\n", i, in);
in = (in + 1) % BUFFER_SIZE;
sem_post(&mutex);
sem_post(&full);
sleep(1);
}
pthread_exit(NULL);
}
void *consumer(void *arg) {
int i;
for (i = 0; i < 20; i++) {
sem_wait(&full);
sem_wait(&mutex);
int item = buffer[out];
printf("Consumer consumed item %d from index %d\n", item, out);
out = (out + 1) % BUFFER_SIZE;
sem_post(&mutex);
sem_post(&empty);
sleep(1);
}
pthread_exit(NULL);
}
int main() {
pthread_t producer_thread, consumer_thread;
// Initialize semaphores
sem_init(&mutex, 0, 1);
sem_init(&empty, 0, BUFFER_SIZE);
sem_init(&full, 0, 0);
// Create threads
pthread_create(&producer_thread, NULL, producer, NULL);
pthread_create(&consumer_thread, NULL, consumer, NULL);
// Wait for threads to finish
pthread_join(producer_thread, NULL);
pthread_join(consumer_thread, NULL);
// Clean up semaphores
sem_destroy(&mutex);
sem_destroy(&empty);
sem_destroy(&full);
return 0;
}
```
在这个代码中,我们使用了三个信号量:`mutex`、`empty` 和 `full`。`mutex` 用于保护共享缓冲区,`empty` 用于表示缓冲区中空余的槽位,`full` 用于表示缓冲区中已经存放的数据项数。
生产者线程首先等待 `empty` 信号量,表示缓冲区中有空余的槽位。然后获得 `mutex` 信号量,将一个新的数据项添加到缓冲区中,并更新 `in` 索引。最后释放 `mutex` 和 `full` 信号量,表示缓冲区中已经存放了一个新的数据项。
消费者线程首先等待 `full` 信号量,表示缓冲区中已经存放了数据项。然后获得 `mutex` 信号量,从缓冲区中取出一个数据项,并更新 `out` 索引。最后释放 `mutex` 和 `empty` 信号量,表示缓冲区中有一个空余的槽位。
注意到我们在生产者和消费者线程中都使用了 `sleep(1)` 函数,这是为了使得程序的输出更加清晰,以便理解。在实际的生产环境中,这些线程需要按照一定的速率进行操作,并且需要使用更加高效的同步方法来保证正确性和性能。
相关推荐
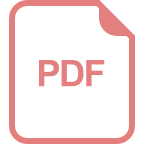
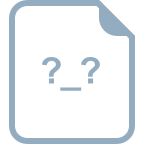
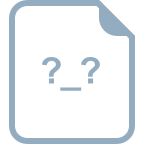














