C++实现一个函数,函数入参是一个string类型的文件夹路径,函数实现删除该文件夹下的所有文件
时间: 2024-02-17 16:00:57 浏览: 13
可以使用以下的C++代码实现该功能:
```cpp
#include <iostream>
#include <string>
#include <filesystem>
void deleteFolder(const std::string& folderPath)
{
std::filesystem::path path(folderPath);
// 如果文件夹不存在或者不是一个文件夹,直接返回
if (!std::filesystem::exists(path) || !std::filesystem::is_directory(path))
{
return;
}
// 遍历文件夹中的所有文件和子文件夹
for (const auto& entry : std::filesystem::recursive_directory_iterator(path))
{
// 如果是一个文件,直接删除
if (std::filesystem::is_regular_file(entry.path()))
{
std::filesystem::remove(entry.path());
}
// 如果是一个文件夹,递归删除
else if (std::filesystem::is_directory(entry.path()))
{
std::filesystem::remove_all(entry.path());
}
}
}
int main()
{
std::string folderPath = "C:\\TestFolder";
deleteFolder(folderPath);
return 0;
}
```
这段代码使用了C++17中引入的std::filesystem库,可以很方便地操作文件和文件夹。函数deleteFolder接受一个文件夹路径作为参数,首先判断该路径是否存在并且是一个文件夹,如果不是则直接返回。然后使用std::filesystem::recursive_directory_iterator遍历该文件夹下的所有文件和子文件夹,对于每一个文件和文件夹进行判断,如果是一个文件直接删除,如果是一个文件夹则递归删除。最后,调用该函数即可删除指定路径下的所有文件。
相关推荐
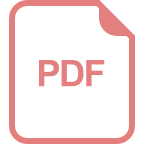
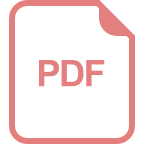
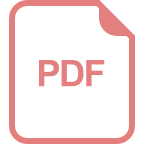














