5、现有一函数: bool SearchFiles(string strFolderPath, vector<string> &vecFilePaths, vector<string> &vecChildFolderPaths); 其功能是在由strFolderPath指定的文件夹路径中,查找文件和子文件夹;文件和子文件夹的绝对路径,将分别存储在vecFilePaths和vecChildFolderPaths;当文件及子文件都不存在时,返回false,其他情况返回true。该函数经过验证,执行结果符合预期,不会发生异常。 请编写一个函数,形式如下: int FindFiles(string strFolderPath, vector<string> &vecFilePaths); 需满足以下要求: 1. 在由strFolderPath指定的文件夹及其各级子文件夹中查找文件,并将所有文件的绝对路径记录在vecFilePaths中; 2. 返回找到的文件个数; 3. 使用递归实现。
时间: 2024-03-16 17:44:23 浏览: 78
下面是实现代码:
```c++
#include <iostream>
#include <vector>
#include <string>
#include <filesystem>
using namespace std;
namespace fs = std::filesystem;
bool SearchFiles(string strFolderPath, vector<string> &vecFilePaths, vector<string> &vecChildFolderPaths) {
bool bFound = false;
for (auto &entry : fs::directory_iterator(strFolderPath)) {
if (entry.is_directory()) {
vecChildFolderPaths.push_back(entry.path().string());
if (SearchFiles(entry.path().string(), vecFilePaths, vecChildFolderPaths)) {
bFound = true;
}
} else {
vecFilePaths.push_back(entry.path().string());
bFound = true;
}
}
return bFound;
}
int FindFiles(string strFolderPath, vector<string> &vecFilePaths) {
vector<string> vecChildFolderPaths;
if (!SearchFiles(strFolderPath, vecFilePaths, vecChildFolderPaths)) {
return 0;
}
return vecFilePaths.size();
}
int main() {
vector<string> vecFilePaths;
int nCount = FindFiles("C:/test", vecFilePaths);
cout << "Found " << nCount << " files:" << endl;
for (auto &path : vecFilePaths) {
cout << path << endl;
}
return 0;
}
```
该函数使用递归实现,在每个文件夹中遍历文件和子文件夹,如果找到文件则将其绝对路径记录在vecFilePaths中,如果找到子文件夹则将其绝对路径记录在vecChildFolderPaths中并递归查找。如果最终没有找到任何文件,则返回0,否则返回找到的文件个数。
阅读全文
相关推荐
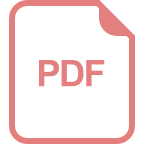
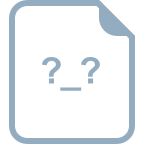
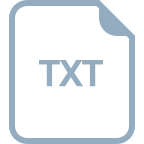

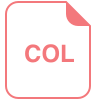
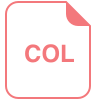
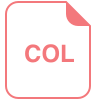
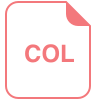
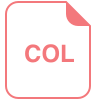
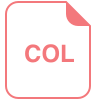

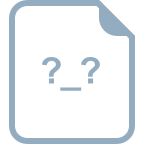
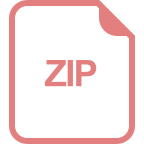
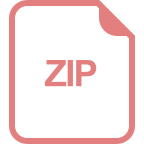
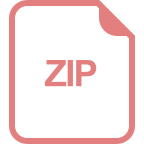
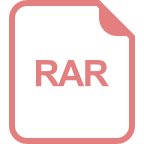
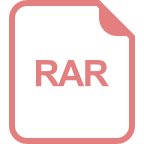
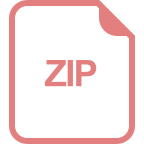