matplotlib画一个折线图
时间: 2023-10-14 18:29:37 浏览: 48
好的,以下是使用Python中的matplotlib库画折线图的示例代码:
```python
import matplotlib.pyplot as plt
# x轴数据
x = [1, 2, 3, 4, 5]
# y轴数据
y = [2, 4, 5, 3, 6]
# 绘制折线图
plt.plot(x, y)
# 设置横轴和纵轴标签
plt.xlabel('x轴')
plt.ylabel('y轴')
# 设置图表标题
plt.title('折线图')
# 显示图表
plt.show()
```
希望这能帮到你。如果你有其他问题,可以随时问我哦。
相关问题
matplotlib画多条折线图
要在 matplotlib 中画多条折线图,可以使用 plt.plot() 函数并传入不同的数据。以下是一个示例代码,其中包含三条折线图:
```python
import matplotlib.pyplot as plt
import numpy as np
# 生成随机数据
x = np.linspace(0, 10, 100)
y1 = np.sin(x)
y2 = np.cos(x)
y3 = np.tan(x)
# 绘制折线图
plt.plot(x, y1)
plt.plot(x, y2)
plt.plot(x, y3)
# 设置图例和标题
plt.legend(['sin(x)', 'cos(x)', 'tan(x)'])
plt.title('Multiple Line Chart')
# 显示图形
plt.show()
```
在这个示例中,我们使用 numpy 库生成了三个不同的数据集,然后使用 plt.plot() 函数分别绘制了它们。我们还使用 plt.legend() 函数添加了一个图例,并使用 plt.title() 函数设置了标题。最后,我们调用 plt.show() 函数显示图形。
python matplotlib 画两个折线图,在同一个界面里面
好的,可以使用 Matplotlib 库中的 subplot() 函数来实现在同一个界面中画多个子图,其中每个子图对应一个折线图。
示例代码如下:
```python
import matplotlib.pyplot as plt
# 定义 x 轴数据
x = [1, 2, 3, 4, 5]
# 定义 y1 和 y2 轴数据
y1 = [2, 4, 6, 8, 10]
y2 = [1, 3, 5, 7, 9]
# 创建画布,并在画布中创建两个子图
fig, (ax1, ax2) = plt.subplots(1, 2, figsize=(10, 5))
# 在第一个子图中画折线图
ax1.plot(x, y1)
ax1.set_xlabel('X Label')
ax1.set_ylabel('Y1 Label')
ax1.set_title('Line Plot 1')
# 在第二个子图中画折线图
ax2.plot(x, y2)
ax2.set_xlabel('X Label')
ax2.set_ylabel('Y2 Label')
ax2.set_title('Line Plot 2')
# 显示图形
plt.show()
```
在这个示例中,我们先定义了两组 x 轴和 y 轴的数据。然后使用 subplots() 函数创建了一个画布,并在画布中创建了两个子图。接着分别在两个子图中使用 plot() 函数画出了对应的折线图,并设置了 x 轴和 y 轴的标签和标题。最后使用 show() 函数显示了图形。
相关推荐
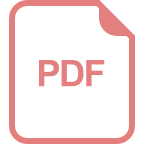
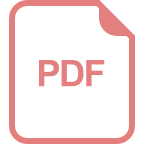
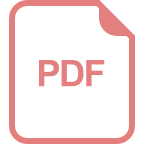












