public GameObject Pan1; public GameObject Pan2; public void bt1() { if (Pan1.activeSelf == false) { Pan1.SetActive(true); Pan2.SetActive(false); } else { Pan1.SetActive(false); } } public void bt2() { if (Pan2.activeSelf == false) { Pan2.SetActive(true); Pan1.SetActive(false); } else { Pan2.SetActive(false); } } Unity 在这个代码上更改 按钮组和panl组 显示隐藏
时间: 2024-02-15 12:27:25 浏览: 131
这段代码是用于在 Unity 中实现按钮和面板的显示和隐藏切换。其中,Pan1 和 Pan2 分别代表两个不同的面板,bt1 和 bt2 是两个不同的按钮。当点击 bt1 按钮时,如果 Pan1 面板当前为隐藏状态,就将其显示出来,并将 Pan2 面板隐藏;如果 Pan1 面板当前为显示状态,就将其隐藏。当点击 bt2 按钮时,同理。这样就可以实现通过点击不同的按钮来控制不同的面板的显示和隐藏。
相关问题
public GameObject Pan1; public GameObject Imag1; public GameObject Pan2; public GameObject Imag2; public GameObject Pan3; public GameObject Imag3; public GameObject Pan4; public GameObject Imag4; public GameObject Pan5; public GameObject Imag5; public void bt1() { if (Pan1.activeSelf == false) { Pan1.SetActive(true); Imag1.SetActive(true); Pan2.SetActive(false); Imag2.SetActive(false); Pan3.SetActive(false); Imag3.SetActive(false); Pan4.SetActive(false); Imag4.SetActive(false); Pan5.SetActive(false); Imag5.SetActive(false); } else { Pan1.SetActive(false); Imag1.SetActive(false); } } public void bt2() { if (Pan2.activeSelf == false) { Pan2.SetActive(true); Imag2.SetActive(true); Pan1.SetActive(false); Imag1.SetActive(false); Pan3.SetActive(false); Imag3.SetActive(false); Pan4.SetActive(false); Imag4.SetActive(false); Pan5.SetActive(false); Imag5.SetActive(false); } else { Pan2.SetActive(false); Imag2.SetActive(false); } } public void bt3() { if (Pan3.activeSelf == false) { Pan3.SetActive(true); Imag3.SetActive(true); Pan1.SetActive(false); Imag1.SetActive(false); Pan2.SetActive(false); Imag2.SetActive(false); Pan4.SetActive(false); Imag4.SetActive(false); Pan5.SetActive(false); Imag5.SetActive(false); } else { Pan3.SetActive(false); Imag3.SetActive(false); } } unity 用更好的方法整理减少这段代码
可以使用数组和循环来简化这段代码,如下所示:
```
public GameObject[] panels;
public GameObject[] images;
public void ShowPanel(int index)
{
for (int i = 0; i < panels.Length; i++)
{
if (i == index)
{
panels[i].SetActive(true);
images[i].SetActive(true);
}
else
{
panels[i].SetActive(false);
images[i].SetActive(false);
}
}
}
```
在这里,我们使用了两个数组来存储面板和图像的引用,并创建了一个函数来显示指定的面板和图像。当函数被调用时,它会遍历所有的面板和图像,如果当前索引等于传递的索引,则设置面板和图像为活动状态,否则将它们设置为非活动状态。这种方法可以大大减少代码行数,使代码更加简洁。
public void bt2() { if (Pan2.activeSelf == false) { Pan2.SetActive(true); Imag2.SetActive(true); Pan1.SetActive(false); Imag1.SetActive(false); Pan3.SetActive(false); Imag3.SetActive(false); Pan4.SetActive(false); Imag4.SetActive(false); Pan5.SetActive(false); Imag5.SetActive(false); } else { Pan2.SetActive(false); Imag2.SetActive(false); } } public void bt3() { if (Pan3.activeSelf == false) { Pan3.SetActive(true); Imag3.SetActive(true); Pan1.SetActive(false); Imag1.SetActive(false); Pan2.SetActive(false); Imag2.SetActive(false); Pan4.SetActive(false); Imag4.SetActive(false); Pan5.SetActive(false); Imag5.SetActive(false); } else { Pan3.SetActive(false); Imag3.SetActive(false); } } unity 用更好的方法整理减少这段代码
可以将重复的代码提取出来,写成一个函数,然后在需要使用的地方调用该函数即可,如下所示:
```
public void ShowPanel(GameObject panel, GameObject image)
{
panel.SetActive(true);
image.SetActive(true);
foreach (Transform child in transform)
{
if (child.gameObject != panel && child.gameObject != image)
{
child.gameObject.SetActive(false);
}
}
}
public void bt2()
{
if (Pan2.activeSelf == false)
{
ShowPanel(Pan2, Imag2);
}
else
{
Pan2.SetActive(false);
Imag2.SetActive(false);
}
}
public void bt3()
{
if (Pan3.activeSelf == false)
{
ShowPanel(Pan3, Imag3);
}
else
{
Pan3.SetActive(false);
Imag3.SetActive(false);
}
}
```
阅读全文
相关推荐
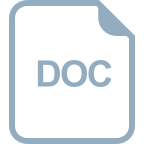
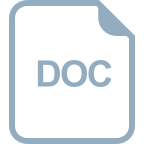
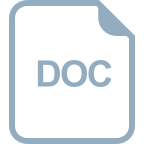
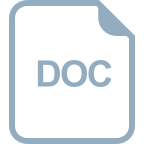
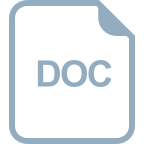
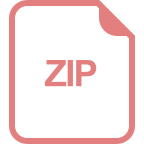
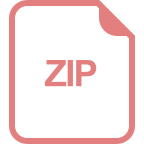
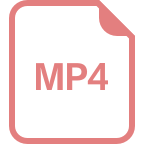
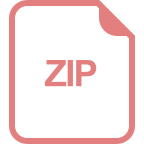
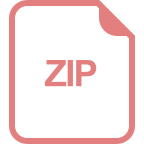
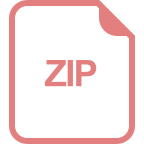
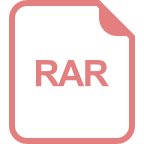
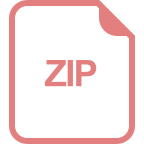
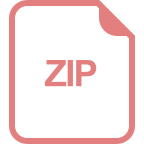