用java实现文本文件的读入、复制、查询和修改的程序
时间: 2023-09-06 11:05:08 浏览: 165
### 回答1:
好的,这是一份用 Java 实现文本文件的读入、复制、查询和修改的程序的示例:
```
import java.io.*;
public class FileOperation {
public static void main(String[] args) {
// 读入文本文件
String filePath = "path/to/file.txt";
String content = readFile(filePath);
System.out.println(content);
// 复制文本文件
String copyPath = "path/to/copy.txt";
copyFile(filePath, copyPath);
// 查询文本文件中的某个字符串
String query = "hello";
boolean exists = searchInFile(filePath, query);
System.out.println(exists);
// 修改文本文件
String oldStr = "old";
String newStr = "new";
replaceInFile(filePath, oldStr, newStr);
}
// 读入文本文件
public static String readFile(String filePath) {
StringBuilder content = new StringBuilder();
try {
BufferedReader reader = new BufferedReader(new FileReader(filePath));
String line;
while ((line = reader.readLine()) != null) {
content.append(line).append("\n");
}
reader.close();
} catch (IOException e) {
e.printStackTrace();
}
return content.toString();
}
// 复制文本文件
public static void copyFile(String sourcePath, String targetPath) {
try {
BufferedReader reader = new BufferedReader(new FileReader(sourcePath));
BufferedWriter writer = new BufferedWriter(new FileWriter(targetPath));
String line;
while ((line = reader.readLine()) != null) {
writer.write(line);
writer.newLine();
}
reader.close();
writer.close();
} catch (IOException e) {
e.printStackTrace();
}
}
// 查询文本文件中的某个字符串
public static boolean searchInFile(String filePath, String query) {
try {
BufferedReader reader = new BufferedReader(new FileReader(filePath));
String line;
while ((line = reader.readLine()) != null) {
if (line.contains(query)) {
reader.close();
return true;
}
}
reader.close();
} catch (IOException e) {
e.printStackTrace();
}
return false;
}
// 修改文本文件
### 回答2:
Java是一种功能强大的编程语言,可以方便地实现文本文件的读入、复制、查询和修改。下面是一个用Java实现这些功能的程序示例。
## 文本文件读入
```java
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class TextFileReader {
public static void main(String[] args) {
try {
BufferedReader reader = new BufferedReader(new FileReader("input.txt"));
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
reader.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
## 文本文件复制
```java
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
public class TextFileCopy {
public static void main(String[] args) {
try {
FileInputStream fis = new FileInputStream("input.txt");
FileOutputStream fos = new FileOutputStream("output.txt");
byte[] buffer = new byte[1024];
int bytesRead;
while ((bytesRead = fis.read(buffer)) != -1) {
fos.write(buffer, 0, bytesRead);
}
fis.close();
fos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
## 文本文件查询
```java
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class TextFileSearch {
public static void main(String[] args) {
try {
BufferedReader reader = new BufferedReader(new FileReader("input.txt"));
String line;
while ((line = reader.readLine()) != null) {
if (line.contains("search_string")) {
System.out.println(line);
}
}
reader.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
## 文本文件修改
```java
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
public class TextFileModification {
public static void main(String[] args) {
try {
BufferedReader reader = new BufferedReader(new FileReader("input.txt"));
BufferedWriter writer = new BufferedWriter(new FileWriter("output.txt"));
String line;
while ((line = reader.readLine()) != null) {
// 对文字进行修改
String modifiedLine = line.replace("old_value", "new_value");
writer.write(modifiedLine + "\n");
}
reader.close();
writer.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
以上就是用Java实现文本文件的读入、复制、查询和修改的程序示例。你可以根据自己的需求对这些示例进行修改和扩展。
### 回答3:
Java可以通过使用FileReader和BufferedReader类来实现文本文件的读入。首先,我们需要创建一个FileReader对象,并将要读取的文件路径传递给它。然后,将FileReader对象传递给BufferedReader对象,以便逐行读取文件内容。使用readLine()方法可以逐行读取文本文件。
复制文件可以使用File类的方法来实现。我们需要创建两个File对象,一个用于指定要复制的源文件的路径,另一个用于指定要创建的副本文件的路径。然后,使用FileInputStream和FileOutputStream类来读取源文件并将内容写入副本文件中。
查询文件可以通过读取文本文件并使用字符串的contains()方法来实现。我们可以使用readLine()方法逐行读取文本文件,并将每一行与要查询的字符串进行比较。
修改文件可以先读取文本文件的内容,然后使用字符串的replace()方法将要修改的部分替换为新的内容。然后,将修改后的内容写回到原文件中。
下面是一个用Java实现文本文件读取、复制、查询和修改的示例程序:
```java
import java.io.*;
public class TextFileUtils {
public static void main(String[] args) {
// 读取文件
String content = readFile("input.txt");
System.out.println("读取的文件内容为:");
System.out.println(content);
// 复制文件
copyFile("input.txt", "copy.txt");
System.out.println("已将文件复制为:copy.txt");
// 查询文件
boolean found = searchFile("input.txt", "example");
if (found) {
System.out.println("在文件中找到了查询字符串。");
} else {
System.out.println("在文件中未找到查询字符串。");
}
// 修改文件
String modifiedContent = modifyFile("input.txt", "example", "sample");
System.out.println("修改后的文件内容为:");
System.out.println(modifiedContent);
}
public static String readFile(String filePath) {
StringBuilder content = new StringBuilder();
try {
BufferedReader reader = new BufferedReader(new FileReader(filePath));
String line;
while ((line = reader.readLine()) != null) {
content.append(line);
content.append("\n");
}
reader.close();
} catch (IOException e) {
e.printStackTrace();
}
return content.toString();
}
public static void copyFile(String sourceFilePath, String destFilePath) {
try {
File sourceFile = new File(sourceFilePath);
File destFile = new File(destFilePath);
FileInputStream inputStream = new FileInputStream(sourceFile);
FileOutputStream outputStream = new FileOutputStream(destFile);
byte[] buffer = new byte[1024];
int length;
while ((length = inputStream.read(buffer)) > 0) {
outputStream.write(buffer, 0, length);
}
inputStream.close();
outputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
public static boolean searchFile(String filePath, String query) {
try {
BufferedReader reader = new BufferedReader(new FileReader(filePath));
String line;
while ((line = reader.readLine()) != null) {
if (line.contains(query)) {
reader.close();
return true;
}
}
reader.close();
} catch (IOException e) {
e.printStackTrace();
}
return false;
}
public static String modifyFile(String filePath, String target, String replacement) {
StringBuilder content = new StringBuilder();
try {
BufferedReader reader = new BufferedReader(new FileReader(filePath));
String line;
while ((line = reader.readLine()) != null) {
line = line.replace(target, replacement);
content.append(line);
content.append("\n");
}
reader.close();
} catch (IOException e) {
e.printStackTrace();
}
return content.toString();
}
}
```
以上程序是一个简单的示例,实现了文件的读取、复制、查询和修改功能。运行时需要确保输入文件存在,并替换`input.txt`为你要操作的文件名。
阅读全文
相关推荐
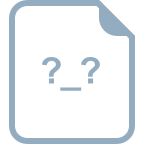


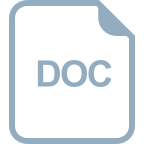
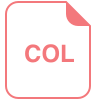
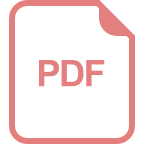
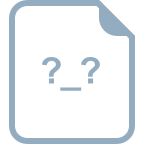
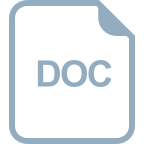
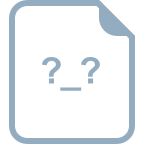
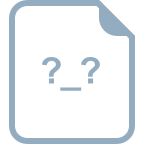
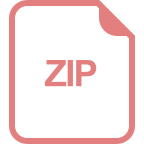
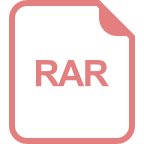
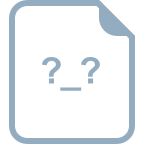
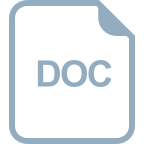
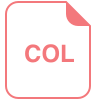
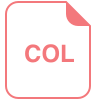
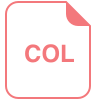