python从两个绝对路径的文件集合获取相对路径的文件集合
时间: 2024-03-01 13:51:47 浏览: 30
可以使用Python的os模块中的os.path.relpath()函数来获取两个绝对路径的文件集合之间的相对路径集合。
示例代码:
```python
import os
# 两个绝对路径的文件集合
abs_set1 = ['/home/user/documents/file1.txt', '/home/user/documents/file2.txt']
abs_set2 = ['/home/user/downloads/file3.txt', '/home/user/downloads/file4.txt']
# 获取相对路径的文件集合
rel_set = []
for abs_path2 in abs_set2:
for abs_path1 in abs_set1:
rel_path = os.path.relpath(abs_path2, os.path.dirname(abs_path1))
rel_set.append(rel_path)
print(rel_set)
```
上述代码将输出`['../downloads/file3.txt', '../downloads/file4.txt']`,表示从`/home/user/documents/`到`/home/user/downloads/`的相对路径为`../downloads`,并且将其应用于`abs_set2`中的文件路径,得到了相对路径的文件集合。
相关问题
用python进行两个文件去重后合并
### 回答1:
可以使用 Python 的 set 和 union 函数来实现两个文件的去重合并。
首先,打开两个文件并读入内容。然后,将每个文件的内容存储在一个 set 中,这样就可以去除重复的行。最后,使用 set 的 union 函数将两个 set 合并起来。
示例代码如下:
```
# 打开文件并读入内容
with open('file1.txt', 'r') as f1:
lines1 = f1.readlines()
with open('file2.txt', 'r') as f2:
lines2 = f2.readlines()
# 将文件内容存储在 set 中,去除重复的行
set1 = set(lines1)
set2 = set(lines2)
# 使用 union 函数将两个 set 合并起来
merged_set = set1.union(set2)
# 将合并后的 set 转换为列表
merged_list = list(merged_set)
# 将合并后的列表写入新文件
with open('merged_file.txt', 'w') as f:
for line in merged_list:
f.write(line)
```
希望这些信息能帮到你!
### 回答2:
使用Python进行两个文件去重后合并可以采用以下步骤:
1. 首先,读取两个文件的内容,可以使用`open()`函数和`readlines()`方法将文件内容读取到列表中。
2. 然后,将两个列表合并成一个新的列表,可以使用`extend()`方法将第二个列表的元素添加到第一个列表中。
3. 接下来,将新的列表进行去重,可以使用`set()`函数将列表转换为集合,由于集合的元素是唯一的,重复的元素会被去除。
4. 最后,将去重后的列表写入到一个新的文件中,可以使用`open()`函数和`writelines()`方法将列表的元素写入文件中。
下面是代码示例:
```python
# 读取两个文件的内容
with open('file1.txt', 'r') as f1, open('file2.txt', 'r') as f2:
lines1 = f1.readlines()
lines2 = f2.readlines()
# 合并两个列表
lines = lines1
lines.extend(lines2)
# 去重
unique_lines = list(set(lines))
# 写入新文件
with open('merged_file.txt', 'w') as f:
f.writelines(unique_lines)
```
以上代码假设要合并的文件为`file1.txt`和`file2.txt`,合并后的结果会写入到`merged_file.txt`中。请根据实际情况修改文件名和文件路径。
### 回答3:
使用Python进行两个文件去重以后合并的一种方法是:
首先,我们需要打开两个文件,分别读取它们的内容。假设这两个文件分别为file1.txt和file2.txt,在当前目录下。
```python
with open("file1.txt", "r") as file1, open("file2.txt", "r") as file2:
content1 = file1.read()
content2 = file2.read()
```
接下来,我们将两个文件的内容进行去重,即去掉重复的行。
```python
lines1 = set(content1.split("\n"))
lines2 = set(content2.split("\n"))
```
然后,我们将去重后的内容合并在一起。
```python
merged_lines = lines1.union(lines2)
```
最后,将合并后的内容写入一个新文件,例如merged_file.txt。
```python
with open("merged_file.txt", "w") as merged_file:
merged_file.write("\n".join(merged_lines))
```
以上代码将两个文件中的内容进行了去重并合并,最终结果保存在merged_file.txt中。
注意:以上代码假设文件中的内容是以换行符分隔的行,如果文件使用其他分隔符,请根据实际情况进行修改。
写个python代码用于对比两个文件夹里的文件
### 回答1:
您好!您可以使用下面的代码来比较两个文件夹里的文件:
```
import os
def compare_files(dir1, dir2):
dir1_files = set(os.listdir(dir1))
dir2_files = set(os.listdir(dir2))
only_in_dir1 = dir1_files - dir2_files
only_in_dir2 = dir2_files - dir1_files
in_both = dir1_files & dir2_files
print("文件仅在目录 1 中:", only_in_dir1)
print("文件仅在目录 2 中:", only_in_dir2)
print("文件在两个目录中都有:", in_both)
compare_files("dir1", "dir2")
```
该代码使用了 Python 的 `os` 库,通过调用 `os.listdir` 函数获取目录中的文件列表。然后使用集合运算(交集、并集等)来比较两个目录中的文件。最后,打印出仅在目录 1 中的文件、仅在目录 2 中的文件和在两个目录中都有的文件。
### 回答2:
可以使用以下Python代码对比两个文件夹中的文件:
import os
def compare_folders(folder1, folder2):
files_1 = os.listdir(folder1)
files_2 = os.listdir(folder2)
common_files = set(files_1) & set(files_2)
only_in_folder1 = set(files_1) - set(files_2)
only_in_folder2 = set(files_2) - set(files_1)
print("共同文件:")
for file in common_files:
print(file)
print("只存在于文件夹1中的文件:")
for file in only_in_folder1:
print(file)
print("只存在于文件夹2中的文件:")
for file in only_in_folder2:
print(file)
# 两个文件夹的路径
folder1_path = "文件夹1的路径"
folder2_path = "文件夹2的路径"
compare_folders(folder1_path, folder2_path)
在上面的代码中,我们首先使用os.listdir()函数获取两个文件夹中的所有文件名。然后使用集合操作符进行比较,找出共同文件,以及只存在于文件夹1或文件夹2中的文件。最后,我们使用循环打印出结果。
请注意,您需要将代码中的"文件夹1的路径"和"文件夹2的路径"替换为实际的文件夹路径。这样,代码就可以正确运行并输出对比结果。
### 回答3:
下面是一个简单的Python代码,用于对比两个文件夹中的文件:
```python
import filecmp
def compare_folders(folder1, folder2):
comparison = filecmp.dircmp(folder1, folder2)
# 比较文件夹中的共同文件
for common_file in comparison.common_files:
file1_path = folder1 + '/' + common_file
file2_path = folder2 + '/' + common_file
if filecmp.cmp(file1_path, file2_path):
print(f"{common_file} 一致")
else:
print(f"{common_file} 不一致")
# 比较仅存在于一个文件夹中的文件
for unique_file in comparison.left_only:
print(f"{unique_file} 仅存在于文件夹1中")
for unique_file in comparison.right_only:
print(f"{unique_file} 仅存在于文件夹2中")
folder1 = '文件夹1的路径'
folder2 = '文件夹2的路径'
compare_folders(folder1, folder2)
```
请将 `'文件夹1的路径'` 和 `'文件夹2的路径'` 替换为具体的文件夹路径,然后运行代码。代码将会比较两个文件夹中的共同文件是否一致,并输出不一致的文件名。同时,还会输出仅存在于一个文件夹中的文件名。
相关推荐
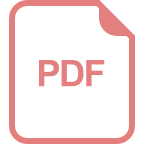
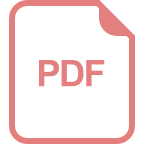
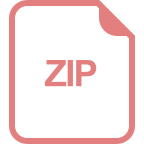












