餐馆菜单管理系统代码是什么,用python
时间: 2024-02-12 09:03:53 浏览: 127
好的,这里提供一个简单的Python代码示例,实现餐馆菜单管理系统的基本功能。代码如下:
```python
# 定义菜品类
class Dish:
def __init__(self, name, price, description):
self.name = name
self.price = price
self.description = description
def __str__(self):
return "{} (${:.2f}): {}".format(self.name, self.price, self.description)
# 定义菜单类
class Menu:
def __init__(self):
self.dishes = []
def add_dish(self, dish):
self.dishes.append(dish)
def remove_dish(self, dish):
self.dishes.remove(dish)
def get_dishes(self):
return sorted(self.dishes, key=lambda x: x.price)
# 定义顾客类
class Customer:
def __init__(self, name):
self.name = name
self.order = {}
def add_dish(self, dish, quantity):
if dish in self.order:
self.order[dish] += quantity
else:
self.order[dish] = quantity
def remove_dish(self, dish):
del self.order[dish]
def get_order(self):
return self.order
def get_total_price(self):
return sum(dish.price * quantity for dish, quantity in self.order.items())
# 初始化菜单
menu = Menu()
menu.add_dish(Dish("Beef Noodle", 10.99, "Hand-pulled noodles with braised beef"))
menu.add_dish(Dish("Hotpot", 20.99, "Spicy hotpot with various ingredients"))
menu.add_dish(Dish("Fried Rice", 8.99, "Fried rice with egg, green onion and soy sauce"))
# 初始化顾客
customer = Customer("John")
# 顾客下单
customer.add_dish(menu.get_dishes()[0], 2)
customer.add_dish(menu.get_dishes()[1], 1)
# 输出菜单和订单
print("Menu:")
for dish in menu.get_dishes():
print(dish)
print("Order:")
for dish, quantity in customer.get_order().items():
print("{} x {} = ${:.2f}".format(dish.name, quantity, dish.price * quantity))
print("Total price: ${:.2f}".format(customer.get_total_price()))
```
在这个示例中,我们定义了菜品类(Dish)、菜单类(Menu)和顾客类(Customer),并通过这些类实现了餐馆菜单管理系统的基本功能。我们通过实例化这些类,并调用相关方法,来添加菜品、下单、结账等操作。当然,这个示例只是一个简单的示例,实际应用中需要根据实际需求进行修改和扩展。
阅读全文
相关推荐
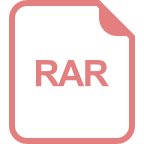



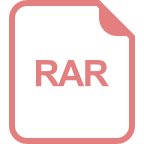
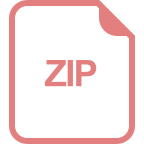
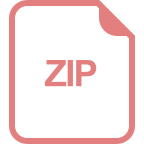
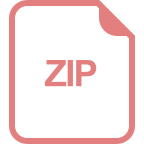
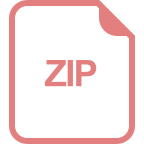
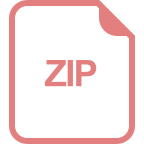
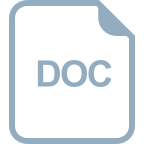
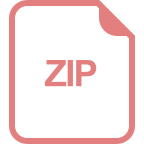
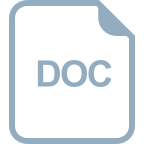
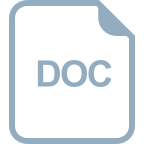
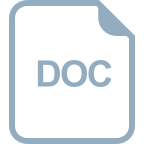
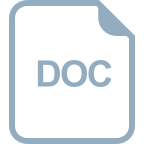

