大一c语言餐馆点菜系统代码
时间: 2023-10-12 15:20:54 浏览: 88
以下是一个简单的餐馆点菜系统的 C 语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_DISHES 10
#define MAX_NAME_LENGTH 20
// 菜品结构体
struct dish {
char name[MAX_NAME_LENGTH];
double price;
};
// 菜单数组
struct dish menu[MAX_DISHES];
// 菜单项数
int num_of_dishes = 0;
// 显示菜单
void show_menu() {
printf("菜单:\n");
for (int i = 0; i < num_of_dishes; i++) {
printf("%d. %s ¥%.2lf\n", i + 1, menu[i].name, menu[i].price);
}
}
// 点菜
void order_dishes() {
int choice;
int counts[MAX_DISHES] = {0};
printf("请输入您要点的菜品编号(以0结束):\n");
while (scanf("%d", &choice) == 1 && choice != 0) {
if (choice < 1 || choice > num_of_dishes) {
printf("输入无效,请重新输入:\n");
continue;
}
counts[choice - 1]++;
}
printf("您点的菜品如下:\n");
double total_price = 0;
for (int i = 0; i < num_of_dishes; i++) {
if (counts[i] > 0) {
printf("%s x %d ¥%.2lf\n", menu[i].name, counts[i], menu[i].price * counts[i]);
total_price += menu[i].price * counts[i];
}
}
printf("总价为:¥%.2lf\n", total_price);
}
int main() {
// 初始化菜单
strcpy(menu[0].name, "宫保鸡丁");
menu[0].price = 28.0;
strcpy(menu[1].name, "鱼香肉丝");
menu[1].price = 32.0;
num_of_dishes = 2;
// 主循环
while (1) {
printf("请选择您要进行的操作:\n");
printf("1. 显示菜单\n");
printf("2. 点菜\n");
printf("0. 退出系统\n");
int choice;
scanf("%d", &choice);
switch (choice) {
case 0:
printf("谢谢使用,再见!\n");
exit(0);
case 1:
show_menu();
break;
case 2:
order_dishes();
break;
default:
printf("输入无效,请重新输入!\n");
break;
}
}
return 0;
}
```
这个程序的功能比较简单,只能显示菜单和点菜,对于一个实际的餐馆点菜系统还需要考虑更多的功能和细节。
阅读全文
相关推荐
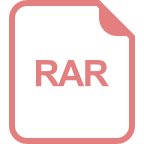
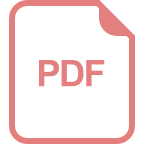

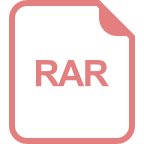
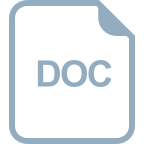
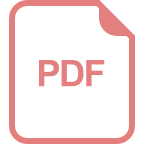
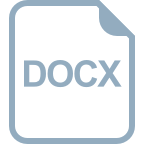
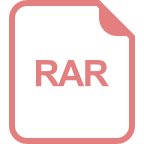
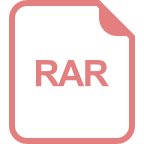