java 批量导出数据到execl
时间: 2024-03-12 08:43:40 浏览: 16
Java 中可以使用 Apache POI 库来实现批量导出数据到 Excel 文件。下面是一个简单的示例代码,假设我们要将一个学生列表导出到 Excel 文件:
```java
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.List;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class ExportToExcel {
public static void export(List<Student> students, String filePath) {
// 创建 Excel 工作簿
XSSFWorkbook workbook = new XSSFWorkbook();
// 创建 Excel 表格
XSSFSheet sheet = workbook.createSheet("学生列表");
// 创建表头
Row headerRow = sheet.createRow(0);
Cell headerCell1 = headerRow.createCell(0);
headerCell1.setCellValue("姓名");
Cell headerCell2 = headerRow.createCell(1);
headerCell2.setCellValue("年龄");
Cell headerCell3 = headerRow.createCell(2);
headerCell3.setCellValue("成绩");
// 填充数据
int rowIndex = 1;
for (Student student : students) {
Row row = sheet.createRow(rowIndex++);
// 姓名
Cell cell1 = row.createCell(0);
cell1.setCellValue(student.getName());
// 年龄
Cell cell2 = row.createCell(1);
cell2.setCellValue(student.getAge());
// 成绩
Cell cell3 = row.createCell(2);
cell3.setCellValue(student.getScore());
}
// 导出文件
try (FileOutputStream outputStream = new FileOutputStream(filePath)) {
workbook.write(outputStream);
System.out.println("导出成功!");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
在上面的代码中,我们通过 `XSSFWorkbook` 类创建了一个 Excel 工作簿,然后通过 `workbook.createSheet` 方法创建了一个 Excel 表格。接着,我们创建了表头,并通过循环遍历学生列表将数据填充到表格中。最后,我们使用 `FileOutputStream` 将 Excel 文件导出到指定的路径。
相关推荐
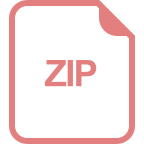
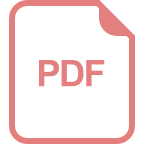
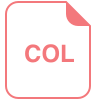
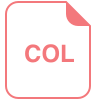
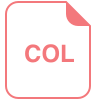
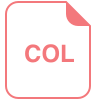
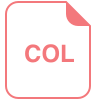









