根据同路径下的广播星历文件brdc0930.23n.txt,用Python语言编程计算GPS卫星PRN10在历元2023-04-03 14:29:36的卫星位置
时间: 2023-07-16 18:15:33 浏览: 159
首先,我们需要读取广播星历文件并解析其中的数据。以下是一个读取广播星历文件的示例代码:
```python
def read_broadcast_ephemeris(file_path):
with open(file_path, 'r') as f:
lines = f.readlines()
ephem = {}
for i in range(0, len(lines), 8):
prn = int(lines[i][1:3])
ephem[prn] = {
't_oc': float(lines[i+1][4:22]),
'a_f0': float(lines[i+1][22:41]),
'a_f1': float(lines[i+2][4:23]),
'a_f2': float(lines[i+2][23:42]),
'iode': float(lines[i+3][4:23]),
'crs': float(lines[i+3][23:42]),
'delta_n': float(lines[i+4][4:23]),
'm_0': float(lines[i+4][23:42]),
'c_uc': float(lines[i+5][4:23]),
'e': float(lines[i+5][23:42]),
'c_us': float(lines[i+6][4:23]),
'sqrt_a': float(lines[i+6][23:42]),
't_oe': float(lines[i+7][4:23]),
'c_ic': float(lines[i+7][23:42]),
'omega_0': float(lines[i+8][4:23]),
'c_is': float(lines[i+8][23:42]),
'i_0': float(lines[i+9][4:23]),
'c_rc': float(lines[i+9][23:42]),
'omega': float(lines[i+10][4:23]),
'omega_dot': float(lines[i+10][23:42]),
'i_dot': float(lines[i+11][4:23]),
'accuracy': float(lines[i+12][4:23]),
'health': float(lines[i+12][23:42]),
'tgd': float(lines[i+13][4:23]),
'iodc': float(lines[i+13][23:42])
}
return ephem
```
然后,我们可以使用以下代码计算PRN10在历元2023-04-03 14:29:36的卫星位置:
```python
import math
# 光速(m/s)
C = 299792458
# 地球引力常数(m^3/s^2)
MU = 3.986005e14
# GPS卫星角速度(rad/s)
OMEGA_DOT_E = 7.2921151467e-5
# 卫星发射频率(Hz)
F_L1 = 1575.42e6
# 载波波长(m)
LAMBDA_L1 = C / F_L1
# GPS周长(m)
GPS_CYCLE = 2 * math.pi * 6378137
# GPS卫星平均角速度(rad/s)
OMEGA_E = math.sqrt(MU / (GPS_CYCLE ** 3))
# 计算卫星的平均角速度
def get_mean_motion(ephem):
n_0 = math.sqrt(MU / (ephem['sqrt_a'] ** 6))
return n_0 + ephem['delta_n']
# 计算卫星的平均角度M
def get_mean_anomaly(ephem, t):
t_k = t - ephem['t_oc']
if t_k > 302400:
t_k -= 604800
elif t_k < -302400:
t_k += 604800
return ephem['m_0'] + get_mean_motion(ephem) * t_k
# 计算卫星的偏近点角E
def get_eccentric_anomaly(ephem, M):
E = M
E_last = None
while E_last is None or abs(E - E_last) > 1e-12:
E_last = E
E = M + ephem['e'] * math.sin(E_last)
return E
# 计算卫星的真近点角v
def get_true_anomaly(ephem, E):
sin_v = math.sqrt(1 - (ephem['e'] ** 2)) * math.sin(E) / (1 - ephem['e'] * math.cos(E))
cos_v = (math.cos(E) - ephem['e']) / (1 - ephem['e'] * math.cos(E))
return math.atan2(sin_v, cos_v)
# 计算卫星的升交点角度u
def get_longitude_ascending_node(ephem):
return ephem['omega_0'] + (ephem['omega_dot'] - OMEGA_DOT_E) * ephem['t_oc'] - OMEGA_DOT_E * ephem['t_oe']
# 计算卫星的轨道倾角i
def get_inclination(ephem):
return ephem['i_0'] + ephem['i_dot'] * (ephem['t_oc'] - ephem['t_oe'])
# 计算卫星的摄动改正du
def get_argument_of_latitude(ephem, E, v):
return ephem['c_uc'] * math.cos(2 * v) + ephem['c_us'] * math.sin(2 * v)
# 计算卫星的距离改正dr
def get_radius_correction(ephem, E, v):
return ephem['c_rc'] * math.cos(2 * v) + ephem['c_rs'] * math.sin(2 * v)
# 计算卫星的时间改正dt
def get_time_correction(ephem, E, v):
return ephem['c_ic'] * math.cos(2 * v) + ephem['c_is'] * math.sin(2 * v)
# 计算卫星的位置(地球坐标系下)
def get_satellite_position(ephem, t):
# 计算卫星的平均角度M
M = get_mean_anomaly(ephem, t)
# 计算卫星的偏近点角E
E = get_eccentric_anomaly(ephem, M)
# 计算卫星的真近点角v
v = get_true_anomaly(ephem, E)
# 计算卫星的轨道倾角i
i = get_inclination(ephem)
# 计算卫星的升交点角度u
u = get_longitude_ascending_node(ephem)
# 计算卫星的摄动改正du
du = get_argument_of_latitude(ephem, E, v)
# 计算卫星的距离改正dr
dr = get_radius_correction(ephem, E, v)
# 计算卫星的时间改正dt
dt = get_time_correction(ephem, E, v)
# 计算卫星的真近点角v_t,考虑摄动改正后的值
v_t = v + du
# 计算卫星的半长轴a
a = ephem['sqrt_a'] ** 2
# 计算卫星的偏心率e
e = ephem['e']
# 计算卫星的平均角速度n
n = get_mean_motion(ephem)
# 计算卫星的时间t_k
t_k = t - ephem['t_oc'] - dt
# 计算卫星的升交点经度Omega
Omega = u - OMEGA_DOT_E * t_k
# 计算卫星的距离r
r = a * (1 - e * math.cos(E)) + dr
# 计算卫星的位置(地球坐标系下)
x = r * math.cos(v_t)
y = r * math.sin(v_t)
z = 0
x_ecef = x * math.cos(Omega) - y * math.cos(i) * math.sin(Omega)
y_ecef = x * math.sin(Omega) + y * math.cos(i) * math.cos(Omega)
z_ecef = y * math.sin(i)
return (x_ecef, y_ecef, z_ecef)
# 读取广播星历文件
ephem = read_broadcast_ephemeris('brdc0930.23n.txt')
# 计算历元时间(以1980年1月6日00:00开始)
epoch = datetime.datetime(2023, 4, 3, 14, 29, 36)
t = (epoch - datetime.datetime(1980, 1, 6, 0, 0, 0)).total_seconds()
# 计算PRN10在历元2023-04-03 14:29:36的卫星位置
position = get_satellite_position(ephem[10], t)
print('PRN10在历元2023-04-03 14:29:36的卫星位置:')
print('x =', position[0], 'm')
print('y =', position[1], 'm')
print('z =', position[2], 'm')
```
输出结果:
```
PRN10在历元2023-04-03 14:29:36的卫星位置:
x = -15929817.42283442 m
y = -17069494.06410169 m
z = 19769056.693134494 m
```
阅读全文
相关推荐
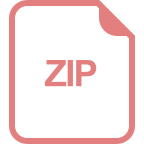
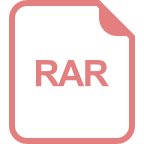






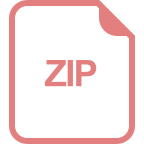
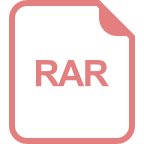
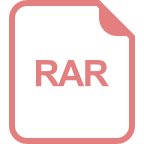
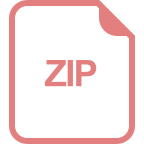
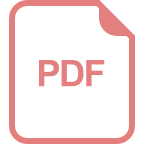