用python 3.9.7 写一个拼图小游戏
时间: 2023-05-13 19:05:42 浏览: 89
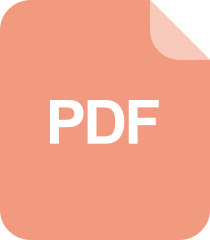
使用Python写一个小游戏
好的,以下是一个简单的拼图小游戏的 Python 代码:
```python
import random
# 定义拼图游戏的类
class PuzzleGame:
def __init__(self, size):
self.size = size
self.board = [[str(i + j * size + 1) for i in range(size)] for j in range(size)]
self.board[size - 1][size - 1] = ' '
# 打印当前的拼图状态
def print_board(self):
for i in range(self.size):
print(' '.join(self.board[i]))
# 移动拼图块
def move(self, direction):
x, y = self.find_space()
if direction == 'up' and y > 0:
self.board[y][x], self.board[y - 1][x] = self.board[y - 1][x], self.board[y][x]
elif direction == 'down' and y < self.size - 1:
self.board[y][x], self.board[y + 1][x] = self.board[y + 1][x], self.board[y][x]
elif direction == 'left' and x > 0:
self.board[y][x], self.board[y][x - 1] = self.board[y][x - 1], self.board[y][x]
elif direction == 'right' and x < self.size - 1:
self.board[y][x], self.board[y][x + 1] = self.board[y][x + 1], self.board[y][x]
# 查找空白块的位置
def find_space(self):
for y in range(self.size):
for x in range(self.size):
if self.board[y][x] == ' ':
return x, y
# 判断是否完成拼图
def is_solved(self):
return str(self) == ' '.join([str(i + 1) for i in range(self.size * self.size - 1)]) + ' '
def __str__(self):
return ' '.join([item for sublist in self.board for item in sublist])
# 主程序
if __name__ == '__main__':
game = PuzzleGame(3)
moves = ['up', 'down', 'left', 'right']
for i in range(100):
game.move(random.choice(moves))
while not game.is_solved():
game.print_board()
direction = input('Enter direction (up, down, left, right): ')
game.move(direction)
print('Congratulations, you solved the puzzle!')
```
这个程序实现了一个 3x3 的拼图游戏,可以通过输入 up、down、left、right 来移动拼图块,直到完成拼图。
阅读全文
相关推荐
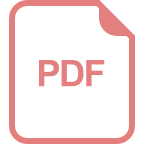

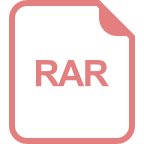













