请用c语言编写一段程序,要求如下:任意输入6个整数,应用选择排序将数据从低到高排序。注意:程序请尽量简洁并给出分析
时间: 2024-05-12 09:16:52 浏览: 9
Sure, I can help you with that. Here's the code in C language using selection sort algorithm to sort 6 integers in ascending order:
```c
#include <stdio.h>
int main() {
int arr[6], i, j, temp, min;
printf("Enter 6 integers: ");
for (i = 0; i < 6; i++) {
scanf("%d", &arr[i]);
}
// Selection sort
for (i = 0; i < 5; i++) {
min = i;
for (j = i + 1; j < 6; j++) {
if (arr[j] < arr[min]) {
min = j;
}
}
temp = arr[i];
arr[i] = arr[min];
arr[min] = temp;
}
// Display sorted array
printf("Sorted array: ");
for (i = 0; i < 6; i++) {
printf("%d ", arr[i]);
}
return 0;
}
```
The program starts by declaring an integer array of size 6 and variables i, j, temp, and min. Then, the user is prompted to enter 6 integers which are stored in the array.
The selection sort algorithm is then used to sort the array in ascending order. In this algorithm, we iterate through each element of the array and find the minimum element from the remaining unsorted elements. We swap the minimum element with the current element, thus placing the minimum element in its correct position. We repeat this process until the whole array is sorted.
Finally, the sorted array is displayed on the screen using a for loop.
I hope this helps! Let me know if you have any further questions or if there's anything else I can assist you with.
相关推荐
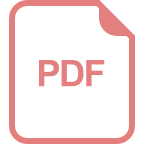
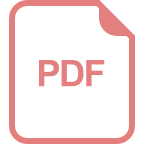
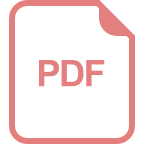














