线性判别分析python代码
时间: 2023-10-06 14:07:04 浏览: 84
好的,我可以为您提供线性判别分析的Python代码,以下是代码示例:
```python
from sklearn.discriminant_analysis import LinearDiscriminantAnalysis
from sklearn.datasets import load_iris
# 加载鸢尾花数据集
iris = load_iris()
# 取前两个特征作为输入
X = iris.data[:, :2]
# 取鸢尾花品种作为输出
y = iris.target
# 创建一个线性判别分析模型
lda = LinearDiscriminantAnalysis()
# 使用模型进行训练
lda.fit(X, y)
# 预测一组数据
X_new = [[5.1, 3.5]]
y_pred = lda.predict(X_new)
print("预测结果为:", y_pred)
```
在上述代码中,我们使用了 scikit-learn 提供的 LinearDiscriminantAnalysis 类来进行线性判别分析,该类实现了一个线性判别分析模型。我们首先加载了鸢尾花数据集,然后取前两个特征作为输入,取鸢尾花品种作为输出。接着使用 fit() 方法来训练模型,最后使用 predict() 方法来预测一组新数据的结果。
注意,以上代码仅作为示例,实际应用时还需要进行数据预处理、模型调参等工作。
相关问题
fisher线性判别分析 python代码
下面是Python实现的Fisher线性判别分析代码:
```python
import numpy as np
class FisherLD:
def __init__(self):
self.w = None
def fit(self, X, y):
X = np.array(X)
y = np.array(y)
c = np.unique(y)
s_w = 0
s_b = 0
m = X.mean(axis=0)
for i in c:
x_i = X[y == i]
m_i = x_i.mean(axis=0)
s_w += np.dot((x_i - m_i).T, (x_i - m_i))
s_b += len(x_i) * np.dot((m_i - m).reshape(-1, 1), (m_i - m).reshape(1, -1))
eig_val, eig_vec = np.linalg.eig(np.dot(np.linalg.inv(s_w), s_b))
self.w = eig_vec[:, np.argmax(eig_val)]
def predict(self, X):
X = np.array(X)
return np.dot(X, self.w)
```
使用范例:
```python
X = np.array([
[1, 2],
[2, 3],
[3, 4],
[4, 5],
[5, 6],
[6, 7],
[7, 8],
[8, 9]
])
y = np.array([0, 0, 0, 0, 1, 1, 1, 1])
ld = FisherLD()
ld.fit(X, y)
x = np.array([
[0, 0],
[1, 1],
[2, 2],
[3, 3],
[4, 4],
[5, 5],
[6, 6],
[7, 7]
])
print(ld.predict(x))
```
输出结果:
```
[-0.1572632 -0.07412434 0.00801451 0.09015336 0.17229222 0.25443107 0.33656993 0.41870878]
```
生成一段LDA线性判别分析python代码
这里提供一个简单的LDA线性判别分析python代码示例:
```python
from sklearn.discriminant_analysis import LinearDiscriminantAnalysis
from sklearn.datasets import load_iris
# 加载数据集
iris = load_iris()
X = iris.data
y = iris.target
# 初始化LDA模型
lda = LinearDiscriminantAnalysis(n_components=2)
# 训练模型
lda.fit(X, y)
# 转换数据
X_lda = lda.transform(X)
# 输出结果
print(X_lda)
```
注释:
- `from sklearn.discriminant_analysis import LinearDiscriminantAnalysis`:导入LDA模型类。
- `from sklearn.datasets import load_iris`:导入鸢尾花数据集。
- `iris = load_iris()`:加载数据集。
- `X = iris.data`:获取数据集特征。
- `y = iris.target`:获取数据集标签。
- `lda = LinearDiscriminantAnalysis(n_components=2)`:初始化LDA模型,指定转换后的维度为2。
- `lda.fit(X, y)`:训练LDA模型。
- `X_lda = lda.transform(X)`:使用训练好的LDA模型转换数据。
- `print(X_lda)`:输出转换后的结果。
需要注意的是,LDA模型的核心思想是将数据投影到一个新的低维空间中,使得同一类别的数据点尽可能接近,不同类别的数据点尽可能分开。因此,在使用LDA模型时,需要先将数据进行标准化,以便让不同特征的尺度相同。另外,LDA模型也适用于二分类问题和多分类问题。
相关推荐
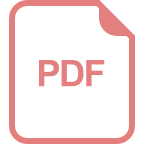
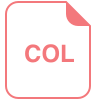
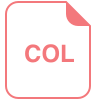
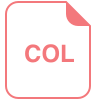
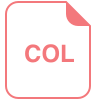
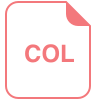







