写一个简单的字符设备驱动
时间: 2024-06-08 07:05:53 浏览: 53
下面是一个简单的字符设备驱动程序的示例,它可以创建一个名为“mychrdev”的字符设备节点,并允许应用程序读取和写入数据:
```
#include <linux/module.h>
#include <linux/fs.h>
#include <linux/uaccess.h>
#define DEVICE_NAME "mychrdev"
#define BUF_LEN 80
static int Major;
static char msg[BUF_LEN];
static char *msg_ptr;
/* Function called when the device is opened */
static int device_open(struct inode *inode, struct file *file)
{
msg_ptr = msg;
return 0;
}
/* Function called when the device is closed */
static int device_release(struct inode *inode, struct file *file)
{
return 0;
}
/* Function called when data is read from the device */
static ssize_t device_read(struct file *filp, char *buffer, size_t length, loff_t *offset)
{
int bytes_read = 0;
while (length && *msg_ptr) {
put_user(*(msg_ptr++), buffer++);
length--;
bytes_read++;
}
return bytes_read;
}
/* Function called when data is written to the device */
static ssize_t device_write(struct file *filp, const char *buffer, size_t length, loff_t *offset)
{
int bytes_written = 0;
while (length && bytes_written < BUF_LEN) {
get_user(msg[bytes_written], buffer++);
length--;
bytes_written++;
}
return bytes_written;
}
/* Define file operations structure */
static struct file_operations fops = {
.read = device_read,
.write = device_write,
.open = device_open,
.release = device_release
};
/* Function called when the module is loaded */
static int __init mychrdev_init(void)
{
Major = register_chrdev(0, DEVICE_NAME, &fops);
if (Major < 0) {
printk(KERN_ALERT "Registering char device failed with %d\n", Major);
return Major;
}
printk(KERN_INFO "Registered char device with major number %d\n", Major);
return 0;
}
/* Function called when the module is unloaded */
static void __exit mychrdev_exit(void)
{
unregister_chrdev(Major, DEVICE_NAME);
printk(KERN_INFO "Unregistered char device\n");
}
module_init(mychrdev_init);
module_exit(mychrdev_exit);
```
在此示例中,我们首先定义了一个设备名称和缓冲区长度。然后定义了设备打开、关闭、读取和写入时需要调用的函数。接下来定义了一个结构体,其中包含了文件操作指针,然后在模块加载时通过 `register_chrdev()` 函数注册该字符设备。
在 `device_read()` 函数中,我们从设备缓冲区中读取数据并将其写入用户空间缓冲区。在 `device_write()` 函数中,我们从用户空间缓冲区中读取数据并将其写入设备缓冲区。在 `device_open()` 函数中,我们初始化缓冲区指针。最后,在模块卸载时,我们通过 `unregister_chrdev()` 函数注销字符设备。
请注意,这只是一个简单的示例程序,实际的设备驱动程序可能需要更多的代码来处理设备的初始化、错误检查、数据校验等。
阅读全文
相关推荐
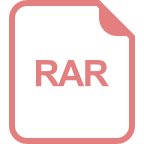
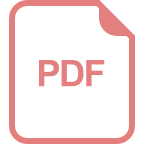
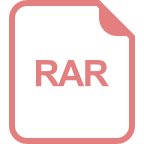
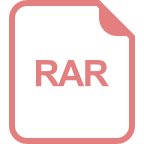
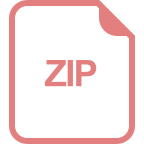
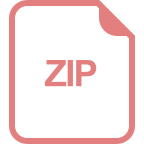
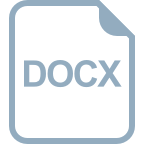
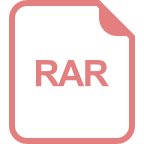
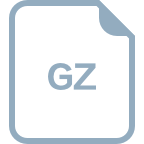
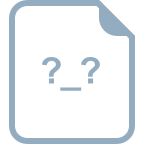