编写一个简单的字符设备驱动程序
时间: 2024-05-14 21:19:31 浏览: 104
好的,我可以帮你解答这个问题。字符设备驱动程序是Linux内核中的一种驱动程序类型,它负责管理字符设备(如串口),使得应用程序可以通过文件IO的方式对设备进行读写操作。下面是一个简单的字符设备驱动程序的框架代码:
```c
#include <linux/module.h>
#include <linux/fs.h>
#include <linux/init.h>
#include <linux/cdev.h>
MODULE_LICENSE("Dual BSD/GPL");
#define DEVICE_NAME "mydevice"
#define CLASS_NAME "mydevice_class"
#define BUFF_SIZE 1024
static int major;
static struct class *myclass = NULL;
static struct device *mydevice = NULL;
static struct cdev c_dev;
static int device_open(struct inode *i, struct file *f)
{
// 在应用程序打开设备文件时调用,可以进行初始化等操作。
// 如果设备被占用,可以返回 -EBUSY。
return 0;
}
static int device_release(struct inode *i, struct file *f)
{
// 在应用程序关闭设备文件时调用,可以进行清理等操作。
return 0;
}
static ssize_t device_read(struct file *f, char __user *buff, size_t len, loff_t *off)
{
// 在应用程序读取设备文件时调用,把设备数据读取到 buff 中。
// 返回值是读取到的字节数,如果已经到文件末尾返回 0。
return 0;
}
static ssize_t device_write(struct file *f, const char __user *buff, size_t len, loff_t *off)
{
// 在应用程序向设备文件中写入数据时调用,把 buff 中的数据写入到设备中。
// 返回值是写入的字节数。
return 0;
}
static struct file_operations f_ops = {
.owner = THIS_MODULE,
.open = device_open,
.release = device_release,
.read = device_read,
.write = device_write
};
static int __init mydevice_init(void)
{
// 注册字符设备驱动程序,在加载模块时调用。
// 这里我们分配一个 cdev 结构体,并注册到内核中。
int ret = alloc_chrdev_region(&major, 0, 1, DEVICE_NAME);
if (ret < 0) {
printk(KERN_ALERT "Failed to allocate device number\n");
return ret;
}
myclass = class_create(THIS_MODULE, CLASS_NAME);
if (IS_ERR(myclass)) {
unregister_chrdev_region(major, 1);
return PTR_ERR(myclass);
}
mydevice = device_create(myclass, NULL, major, NULL, DEVICE_NAME);
if (IS_ERR(mydevice)) {
class_destroy(myclass);
unregister_chrdev_region(major, 1);
return PTR_ERR(mydevice);
}
cdev_init(&c_dev, &f_ops);
ret = cdev_add(&c_dev, major, 1);
if (ret < 0) {
device_destroy(myclass, major);
class_destroy(myclass);
unregister_chrdev_region(major, 1);
return ret;
}
printk(KERN_INFO "Registered a new device with major number %d\n", major);
return 0;
}
static void __exit mydevice_exit(void)
{
// 卸载字符设备驱动程序,在卸载模块时调用。
cdev_del(&c_dev);
device_destroy(myclass, major);
class_destroy(myclass);
unregister_chrdev_region(major, 1);
printk(KERN_INFO "Unregistered the device\n");
}
module_init(mydevice_init);
module_exit(mydevice_exit);
```
这段代码实现了一个名为 `mydevice` 的字符设备驱动程序,提供了 `open`、`release`、`read` 和 `write` 四个驱动接口函数。在加载模块时,会为该设备分配一个主设备号,然后创建设备结点,并将驱动接口函数注册到内核中。在卸载模块时,会删除设备结点以及注销驱动接口函数。
当然,这仅仅是一个最基础的示例,真正的字符设备驱动还需要对设备进行一系列的初始化、卸载、IO操作等。
阅读全文
相关推荐
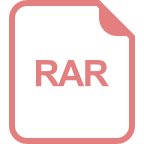
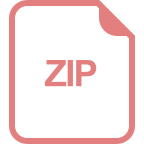
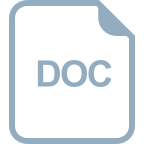
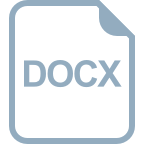
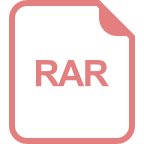
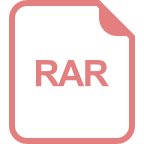
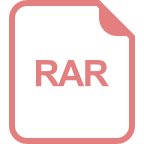
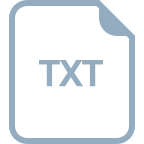
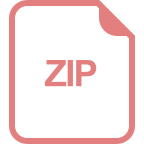
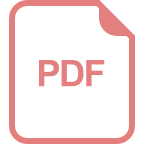
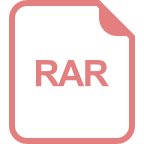
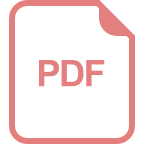
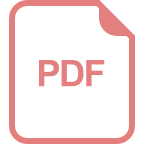
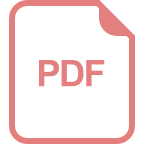
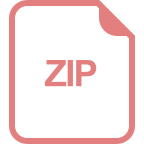