python中zero函数使用
时间: 2023-09-12 18:03:31 浏览: 99
在Python中没有名为“zero”的内置函数,但是你可以使用以下方法来创建一个函数来实现该功能:
```python
def zero(n):
if n == 0:
return True
else:
return False
```
这个函数接受一个参数n,并检查它是否等于0。如果是,则返回True,否则返回False。你可以像这样调用该函数:
```python
print(zero(0)) # 输出 True
print(zero(5)) # 输出 False
```
注意,Python中的整数可以是正数、负数或零。如果你想同时检查一个数字是否等于0或是否为None,你可以使用以下代码:
```python
def is_zero_or_none(n):
if n in (0, None):
return True
else:
return False
```
这个函数使用in关键字检查n是否等于0或None。你可以像这样调用该函数:
```python
print(is_zero_or_none(0)) # 输出 True
print(is_zero_or_none(None)) # 输出 True
print(is_zero_or_none(5)) # 输出 False
```
相关问题
opt函数在Python中的使用方法
opt函数是PyTorch中的优化器函数,用于优化神经网络模型的参数。使用方法如下:
首先需要导入torch.optim模块,然后创建一个优化器对象,指定要优化的参数和优化方法,最后再使用optimizer对象对参数进行更新。示例代码如下:
```python
import torch.optim as optim
# 定义一个神经网络模型
model = nn.Sequential(
nn.Linear(10,20),
nn.ReLU(),
nn.Linear(20,2)
)
# 定义损失函数
criterion = nn.CrossEntropyLoss()
# 定义优化器
optimizer = optim.SGD(model.parameters(), lr=0.01)
# 进行模型训练
for epoch in range(num_epochs):
# 前向传播
output = model(inputs)
loss = criterion(output, labels)
# 反向传播
optimizer.zero_grad()
loss.backward()
# 参数更新
optimizer.step()
```
python 怎么使用ifs函数
如果你想使用 Python 中的 `if` 语句,你可以这样写:
```
if 条件:
# 条件成立时要执行的代码
else:
# 条件不成立时要执行的代码
```
条件可以是任何能够被 Python 解释器评估为 `True` 或 `False` 的表达式。例如:
```
x = 42
if x > 0:
print("x is positive")
```
此代码会输出 `x is positive`,因为 `x > 0` 被评估为 `True`。
同时,你还可以使用多个 `elif` 子句来编写更复杂的逻辑:
```
x = 42
if x < 0:
print("x is negative")
elif x == 0:
print("x is zero")
else:
print("x is positive")
```
这段代码会输出 `x is positive`,因为 `x == 0` 被评估为 `False`,而 `x > 0` 被评估为 `True`。
相关推荐
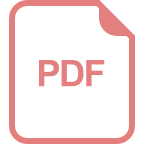
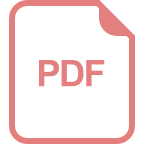
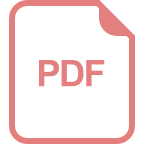
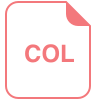
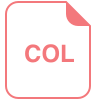
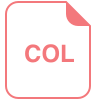
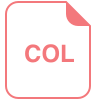
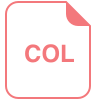







