如何使用Python函数实现图像风格迁移?
时间: 2024-04-16 16:23:27 浏览: 134
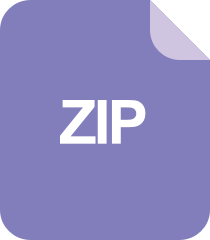
统计学习课程挑战问题,Python实现基于卷积神经网络的图像风格迁移项目源码
图像风格迁移是一种将一张图像的风格应用到另一张图像上的技术。下面是使用Python函数实现图像风格迁移的步骤:
1. 导入所需的模块和库[^2]:
```python
import torch
from PIL import Image
import matplotlib.pyplot as plt
import torchvision.transforms as transforms
import torchvision.models as models
```
2. 加载预训练的VGG-19模型[^1]:
```python
device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
model = models.vgg19(pretrained=True).features
model.to(device).eval()
```
3. 定义图像转换函数:
```python
def image_transform(image_path):
image = Image.open(image_path)
image_transform = transforms.Compose([
transforms.Resize(400),
transforms.ToTensor(),
transforms.Normalize(mean=[0.485, 0.456, 0.406], std=[0.229, 0.224, 0.225])
])
image = image_transform(image).unsqueeze(0)
return image.to(device)
```
4. 加载内容图像和风格图像:
```python
content_image = image_transform("content.jpg")
style_image = image_transform("style.jpg")
```
5. 定义内容损失函数和风格损失函数:
```python
def content_loss(content_features, target_features):
return torch.mean((content_features - target_features) ** 2)
def style_loss(style_features, target_features):
_, c, h, w = style_features.size()
style_features = style_features.view(c, h * w)
target_features = target_features.view(c, h * w)
gram_style = torch.mm(style_features, style_features.t())
gram_target = torch.mm(target_features, target_features.t())
return torch.mean((gram_style - gram_target) ** 2)
```
6. 定义总损失函数:
```python
def total_loss(content_features, style_features, target_features):
content_weight = 1
style_weight = 1000
content_loss_value = content_loss(content_features, target_features)
style_loss_value = style_loss(style_features, target_features)
total_loss = content_weight * content_loss_value + style_weight * style_loss_value
return total_loss
```
7. 进行图像风格迁移:
```python
input_image = content_image.clone().requires_grad_(True).to(device)
optimizer = torch.optim.Adam([input_image], lr=0.01)
num_epochs = 2000
for epoch in range(num_epochs):
optimizer.zero_grad()
input_features = model(input_image)
content_features = model(content_image)
style_features = model(style_image)
loss = total_loss(content_features, style_features, input_features)
loss.backward()
optimizer.step()
if (epoch+1) % 100 == 0:
print(f"Epoch [{epoch+1}/{num_epochs}], Loss: {loss.item():.4f}")
output_image = input_image.detach().squeeze(0).cpu()
output_image = transforms.ToPILImage()(output_image)
output_image.save("output.jpg")
```
这样,你就可以使用Python函数实现图像风格迁移了。
阅读全文
相关推荐
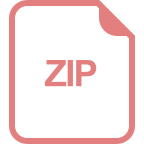
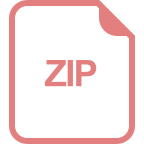
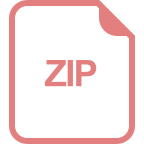
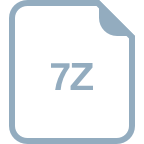
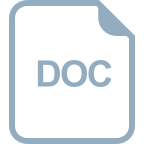
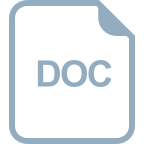
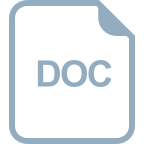
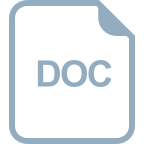
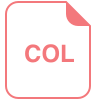
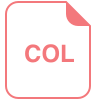
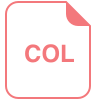
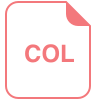
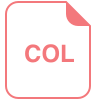




