图像风格迁移python代码
时间: 2023-12-01 15:43:07 浏览: 50
以下是一个基于PyTorch实现的图像风格迁移的Python代码示例:
```python
import torch
import torch.nn as nn
import torch.optim as optim
import torchvision.transforms as transforms
import torchvision.models as models
from PIL import Image
import matplotlib.pyplot as plt
# 定义图像处理函数
loader = transforms.Compose([
transforms.Resize((512, 512)), # 调整图像大小
transforms.ToTensor() # 转换为张量
])
unloader = transforms.ToPILImage() # 将张量转换为图像
# 定义图像加载函数
def image_loader(image_name):
image = Image.open(image_name)
image = loader(image).unsqueeze(0)
return image.to(device, torch.float)
# 定义模型
class ContentLoss(nn.Module):
def __init__(self, target):
super(ContentLoss, self).__init__()
self.target = target.detach()
def forward(self, input):
self.loss = nn.functional.mse_loss(input, self.target)
return input
class StyleLoss(nn.Module):
def __init__(self, target_feature):
super(StyleLoss, self).__init__()
self.target = gram_matrix(target_feature).detach()
def forward(self, input):
G = gram_matrix(input)
self.loss = nn.functional.mse_loss(G, self.target)
return input
def gram_matrix(input):
a, b, c, d = input.size()
features = input.view(a * b, c * d)
G = torch.mm(features, features.t())
return G.div(a * b * c * d)
class Normalization(nn.Module):
def __init__(self, mean, std):
super(Normalization, self).__init__()
self.mean = torch.tensor(mean).view(-1, 1, 1)
self.std = torch.tensor(std).view(-1, 1, 1)
def forward(self, img):
return (img - self.mean) / self.std
# 定义模型
class StyleTransferModel(nn.Module):
def __init__(self, content_img, style_img, cnn=models.vgg19(pretrained=True).features.to(device).eval(),
content_layers=['conv_4'], style_layers=['conv_1', 'conv_2', 'conv_3', 'conv_4', 'conv_5']):
super(StyleTransferModel, self).__init__()
self.content_layers = content_layers
self.style_layers = style_layers
self.content_losses = []
self.style_losses = []
self.model = nn.Sequential(Normalization([0.485, 0.456, 0.406], [0.229, 0.224, 0.225]))
i = 0
for layer in cnn.children():
if isinstance(layer, nn.Conv2d):
i += 1
name = 'conv_{}'.format(i)
elif isinstance(layer, nn.ReLU):
name = 'relu_{}'.format(i)
layer = nn.ReLU(inplace=False)
elif isinstance(layer, nn.MaxPool2d):
name = 'pool_{}'.format(i)
elif isinstance(layer, nn.BatchNorm2d):
name = 'bn_{}'.format(i)
else:
raise RuntimeError('Unrecognized layer: {}'.format(layer.__class__.__name__))
self.model.add_module(name, layer)
if name in content_layers:
target = self.model(content_img).detach()
content_loss = ContentLoss(target)
self.model.add_module("content_loss_{}".format(i), content_loss)
self.content_losses.append(content_loss)
if name in style_layers:
target_feature = self.model(style_img).detach()
style_loss = StyleLoss(target_feature)
self.model.add_module("style_loss_{}".format(i), style_loss)
self.style_losses.append(style_loss)
for i in range(len(self.model) - 1, -1, -1):
if isinstance(self.model[i], ContentLoss) or isinstance(self.model[i], StyleLoss):
break
self.model = self.model[:(i + 1)]
def forward(self, x):
return self.model(x)
# 定义图像风格迁移函数
def style_transfer(content_img_path, style_img_path, num_steps=300,
style_weight=100000, content_weight=1):
content_img = image_loader(content_img_path)
style_img = image_loader(style_img_path)
input_img = content_img.clone()
optimizer = optim.LBFGS([input_img.requires_grad_()])
model = StyleTransferModel(content_img, style_img).to(device)
print('Start style transfer...')
run = [0]
while run[0] <= num_steps:
def closure():
input_img.data.clamp_(0, 1)
optimizer.zero_grad()
model(input_img)
style_score = 0
content_score = 0
for sl in model.style_losses:
style_score += sl.loss
for cl in model.content_losses:
content_score += cl.loss
style_score *= style_weight
content_score *= content_weight
loss = style_score + content_score
loss.backward()
run[0] += 1
if run[0] % 50 == 0:
print("run {}:".format(run))
print('Style Loss : {:4f} Content Loss: {:4f}'.format(
style_score.item(), content_score.item()))
print()
return style_score + content_score
optimizer.step(closure)
input_img.data.clamp_(0, 1)
return input_img
# 定义图像显示函数
def imshow(tensor, title=None):
image = tensor.cpu().clone()
image = image.squeeze(0)
image = unloader(image)
plt.imshow(image)
if title is not None:
plt.title(title)
plt.pause(0.001)
# 设置设备
device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
# 运行图像风格迁移
output = style_transfer('content.jpg', 'style.jpg')
# 显示结果
plt.figure()
imshow(output, title='Output Image')
# 保存结果
output_img = unloader(output.cpu().clone().squeeze(0))
output_img.save('output.jpg')
```
相关推荐
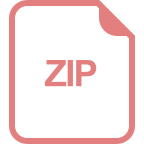
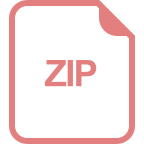














