用eclipse写一个基于java,swing,和mysql的简单的人事管理系统。本设计包括的功能模块如下: (1)基本信息管理 管理人员的基本信息,包括:增加员工信息、修改基础信息、删除员工信息、查询基础信息;维护部门信息,包括:增加、修改、删除、查询等操作。 (2)人员调动管理 管理人员的调动情况,记录人员的调动历史并提供查询功能。 (3)人员考核管理 管理人员的考核情况,记录人员的考核历史并提供查询功能。 (4)劳资管理 管理人员的劳资分配情况,记录人员的劳资更改历史并提供查询功能。请写出程序代码。
时间: 2024-03-11 10:50:32 浏览: 183
由于篇幅限制,我无法在这里为您提供完整的程序代码,但我可以给您提供一个整体的思路和一些代码示例来帮助您完成这个项目。
1. 建立数据库连接
首先,在Java代码中,你需要连接到MySQL数据库。你可以使用JDBC API来连接到数据库。以下是一个建立数据库连接的示例代码:
```
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class DBConnection {
private static final String URL = "jdbc:mysql://localhost:3306/employee";
private static final String USER = "root";
private static final String PASSWORD = "123456";
private static Connection conn = null;
public static Connection getConnection() {
try {
Class.forName("com.mysql.jdbc.Driver");
conn = DriverManager.getConnection(URL, USER, PASSWORD);
} catch (ClassNotFoundException e) {
e.printStackTrace();
} catch (SQLException e) {
e.printStackTrace();
}
return conn;
}
}
```
2. 实现基本信息管理
在基本信息管理模块中,我们需要实现员工信息的增加、修改、删除和查询操作,以及部门信息的维护。以下是一些示例代码:
增加员工信息:
```
public void addEmployee(Employee employee) {
Connection conn = DBConnection.getConnection();
String sql = "INSERT INTO employee(name, gender, position, salary) VALUES (?, ?, ?, ?)";
try {
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setString(1, employee.getName());
pstmt.setString(2, employee.getGender());
pstmt.setString(3, employee.getPosition());
pstmt.setInt(4, employee.getSalary());
pstmt.executeUpdate();
pstmt.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
```
修改员工信息:
```
public void updateEmployee(Employee employee) {
Connection conn = DBConnection.getConnection();
String sql = "UPDATE employee SET name=?, gender=?, position=?, salary=? WHERE id=?";
try {
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setString(1, employee.getName());
pstmt.setString(2, employee.getGender());
pstmt.setString(3, employee.getPosition());
pstmt.setInt(4, employee.getSalary());
pstmt.setInt(5, employee.getId());
pstmt.executeUpdate();
pstmt.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
```
删除员工信息:
```
public void deleteEmployee(int id) {
Connection conn = DBConnection.getConnection();
String sql = "DELETE FROM employee WHERE id=?";
try {
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, id);
pstmt.executeUpdate();
pstmt.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
```
查询员工信息:
```
public Employee getEmployeeById(int id) {
Connection conn = DBConnection.getConnection();
String sql = "SELECT * FROM employee WHERE id=?";
Employee employee = null;
try {
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, id);
ResultSet rs = pstmt.executeQuery();
if (rs.next()) {
employee = new Employee();
employee.setId(rs.getInt("id"));
employee.setName(rs.getString("name"));
employee.setGender(rs.getString("gender"));
employee.setPosition(rs.getString("position"));
employee.setSalary(rs.getInt("salary"));
}
rs.close();
pstmt.close();
} catch (SQLException e) {
e.printStackTrace();
}
return employee;
}
```
增加部门信息:
```
public void addDepartment(Department department) {
Connection conn = DBConnection.getConnection();
String sql = "INSERT INTO department(name, description) VALUES (?, ?)";
try {
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setString(1, department.getName());
pstmt.setString(2, department.getDescription());
pstmt.executeUpdate();
pstmt.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
```
3. 实现人员调动管理、人员考核管理和劳资管理
在这三个模块中,我们需要创建独立的表来存储相应的信息,并实现增加、修改、删除和查询操作。以下是一些示例代码:
增加调动记录:
```
public void addTransferRecord(TransferRecord record) {
Connection conn = DBConnection.getConnection();
String sql = "INSERT INTO transfer_record(employee_id, from_department, to_department, transfer_date) VALUES (?, ?, ?, ?)";
try {
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, record.getEmployeeId());
pstmt.setString(2, record.getFromDepartment());
pstmt.setString(3, record.getToDepartment());
pstmt.setDate(4, new java.sql.Date(record.getTransferDate().getTime()));
pstmt.executeUpdate();
pstmt.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
```
修改考核记录:
```
public void updateAssessmentRecord(AssessmentRecord record) {
Connection conn = DBConnection.getConnection();
String sql = "UPDATE assessment_record SET employee_id=?, assessment_date=?, score=?, comment=? WHERE id=?";
try {
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, record.getEmployeeId());
pstmt.setDate(2, new java.sql.Date(record.getAssessmentDate().getTime()));
pstmt.setInt(3, record.getScore());
pstmt.setString(4, record.getComment());
pstmt.setInt(5, record.getId());
pstmt.executeUpdate();
pstmt.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
```
删除劳资记录:
```
public void deleteSalaryRecord(int id) {
Connection conn = DBConnection.getConnection();
String sql = "DELETE FROM salary_record WHERE id=?";
try {
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, id);
pstmt.executeUpdate();
pstmt.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
```
查询劳资记录:
```
public List<SalaryRecord> getSalaryRecordsByEmployeeId(int employeeId) {
Connection conn = DBConnection.getConnection();
String sql = "SELECT * FROM salary_record WHERE employee_id=?";
List<SalaryRecord> records = new ArrayList<SalaryRecord>();
try {
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, employeeId);
ResultSet rs = pstmt.executeQuery();
while (rs.next()) {
SalaryRecord record = new SalaryRecord();
record.setId(rs.getInt("id"));
record.setEmployeeId(rs.getInt("employee_id"));
record.setDate(rs.getDate("date"));
record.setSalary(rs.getInt("salary"));
records.add(record);
}
rs.close();
pstmt.close();
} catch (SQLException e) {
e.printStackTrace();
}
return records;
}
```
4. 创建用户界面
最后,你可以使用Swing GUI库来创建一个用户界面,使用户可以通过图形界面来执行上述操作。以下是一些示例代码:
```
public class MainFrame extends JFrame {
private JTabbedPane tabbedPane;
private EmployeePanel employeePanel;
private DepartmentPanel departmentPanel;
private TransferRecordPanel transferRecordPanel;
private AssessmentRecordPanel assessmentRecordPanel;
private SalaryRecordPanel salaryRecordPanel;
public MainFrame() {
setTitle("人事管理系统");
setSize(800, 600);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
tabbedPane = new JTabbedPane();
employeePanel = new EmployeePanel();
departmentPanel = new DepartmentPanel();
transferRecordPanel = new TransferRecordPanel();
assessmentRecordPanel = new AssessmentRecordPanel();
salaryRecordPanel = new SalaryRecordPanel();
tabbedPane.addTab("员工信息", employeePanel);
tabbedPane.addTab("部门信息", departmentPanel);
tabbedPane.addTab("人员调动", transferRecordPanel);
tabbedPane.addTab("人员考核", assessmentRecordPanel);
tabbedPane.addTab("劳资管理", salaryRecordPanel);
getContentPane().add(tabbedPane);
setVisible(true);
}
}
```
以上是一个整体的思路和一些代码示例,希望对您有所帮助!如果您需要更详细的代码示例或者对具体实现有疑问,可以在评论区留言,我会尽力回答您的问题。
阅读全文
相关推荐
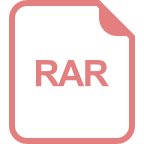
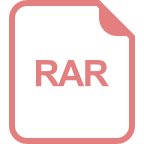
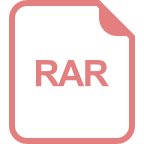
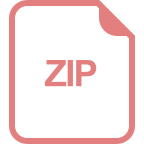
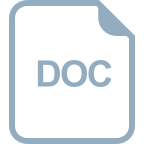
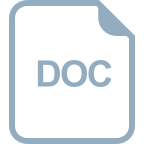

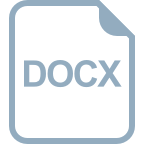
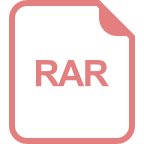
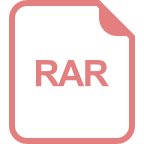
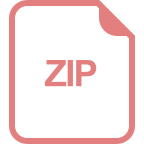
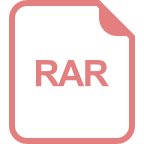
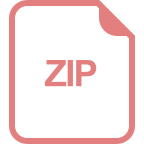
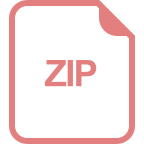
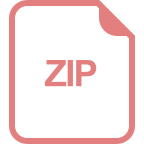
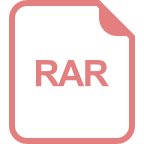
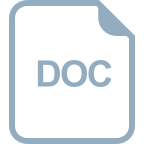
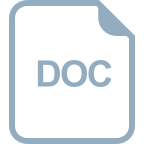
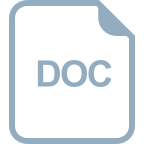
