python 将series添加到dataframe中
时间: 2024-05-15 18:15:27 浏览: 103
可以使用DataFrame的“[ ]”操作符来添加Series到DataFrame中。
假设有一个DataFrame df和一个Series s,可以将s添加到df中的新列中,如下所示:
```
import pandas as pd
# 创建DataFrame
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
# 创建Series
s = pd.Series([7, 8, 9])
# 将Series添加到DataFrame中,作为新列C
df['C'] = s
print(df)
```
输出:
```
A B C
0 1 4 7
1 2 5 8
2 3 6 9
```
也可以将Series添加到DataFrame中的现有列中,如下所示:
```
import pandas as pd
# 创建DataFrame
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6], 'C': [7, 8, 9]})
# 创建Series
s = pd.Series([10, 11, 12])
# 将Series添加到DataFrame中的现有列B中
df['B'] = s
print(df)
```
输出:
```
A B C
0 1 10 7
1 2 11 8
2 3 12 9
```
相关问题
Python中将series转换成dataframe
在Python的Pandas库中,Series是一种一维的数据结构,而DataFrame则是二维表格型的数据结构。如果你有一个Series,并希望将其转换为DataFrame,通常是因为你需要对数据进行更多的操作,如添加列或行,或者利用DataFrame自带的内置函数。
以下是如何将Series转换为DataFrame的基本步骤:
1. 创建一个Series:
```python
import pandas as pd
s = pd.Series(data=[1, 2, 3, 4], index=['a', 'b', 'c', 'd'])
```
2. 使用`pd.DataFrame()`函数并将Series作为参数传入:
```python
df = pd.DataFrame(s)
```
此时,如果Series的索引和DataFrame的默认索引不匹配,你可以通过`index`参数指定索引:
```python
df = pd.DataFrame(s, index=['e', 'f', 'g', 'h'])
```
或者使用`reset_index()`方法手动设置:
```python
df = s.reset_index(drop=True) # 如果你想保留唯一索引,drop=True会移除默认的0,1,2...索引
```
3. DataFrame现在包含了原Series的所有数据,你可以像处理DataFrame一样进行操作了。
python怎么将series转换成DataFrame
可以使用pandas库中的to_frame()方法将series转换成dataframe,示例代码如下:
```python
import pandas as pd
# 创建一个series
s = pd.Series([1, 2, 3, 4, 5])
# 将series转换成dataframe
df = s.to_frame()
# 打印结果
print(df)
```
输出结果如下:
```
0
0 1
1 2
2 3
3 4
4 5
```
阅读全文
相关推荐
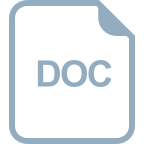
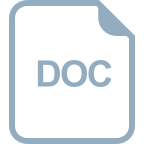
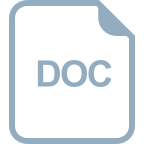
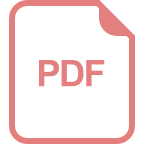












