vue实现当用户点击quill-editor工具栏中的“添加链接”按钮时,将弹出一个提示框要求输入 URL如果用户输入 URL 并单击“确定”,则 Quill 将在当前光标位置添加一个链接。
时间: 2023-11-28 16:47:29 浏览: 23
可以通过以下步骤实现:
1. 在 Vue 组件中引入 Quill 和 Quill 的链接模块
```js
import Quill from 'quill'
import 'quill/dist/quill.core.css'
import 'quill/dist/quill.snow.css'
import 'quill/dist/quill.bubble.css'
import Link from 'quill/modules/link'
```
2. 在组件的 mounted 钩子函数中初始化 Quill 编辑器,并启用链接模块
```js
mounted () {
this.quill = new Quill(this.$refs.editor, {
modules: {
toolbar: [
// 添加链接按钮
['link', 'image', 'video']
],
// 启用链接模块
link: true
},
theme: 'snow'
})
// 注册监听链接按钮点击事件
this.quill.getModule('toolbar').handlers.link = this.showLinkPrompt
// 注册链接模块的保存链接事件
this.quill.getModule('link').setAttrs = attrs => {
if (this.linkPromptDeferred) {
this.linkPromptDeferred.resolve(attrs)
this.linkPromptDeferred = null
}
}
},
```
3. 编写 showLinkPrompt 方法,用于弹出提示框并等待用户输入链接地址
```js
showLinkPrompt () {
// 创建一个 Deferred 对象,用于等待用户输入链接地址
this.linkPromptDeferred = new Deferred()
// 弹出提示框
const url = prompt('请输入链接地址:')
// 如果用户输入了链接地址,则保存链接
if (url) {
this.linkPromptDeferred.resolve({ href: url })
this.linkPromptDeferred = null
}
// 返回 Deferred 对象的 Promise
return this.linkPromptDeferred.promise
}
```
4. 定义一个 Deferred 类,用于封装 Promise,以便等待用户输入链接地址
```js
class Deferred {
constructor () {
this.promise = new Promise((resolve, reject) => {
this.resolve = resolve
this.reject = reject
})
}
}
```
5. 在组件的 beforeDestroy 钩子函数中销毁 Quill 编辑器
```js
beforeDestroy () {
if (this.quill) {
this.quill.destroy()
this.quill = null
}
}
```
完整的代码示例:
```vue
<template>
<div>
<div ref="editor"></div>
</div>
</template>
<script>
import Quill from 'quill'
import 'quill/dist/quill.core.css'
import 'quill/dist/quill.snow.css'
import 'quill/dist/quill.bubble.css'
import Link from 'quill/modules/link'
class Deferred {
constructor () {
this.promise = new Promise((resolve, reject) => {
this.resolve = resolve
this.reject = reject
})
}
}
export default {
mounted () {
this.quill = new Quill(this.$refs.editor, {
modules: {
toolbar: [
// 添加链接按钮
['link', 'image', 'video']
],
// 启用链接模块
link: true
},
theme: 'snow'
})
// 注册监听链接按钮点击事件
this.quill.getModule('toolbar').handlers.link = this.showLinkPrompt
// 注册链接模块的保存链接事件
this.quill.getModule('link').setAttrs = attrs => {
if (this.linkPromptDeferred) {
this.linkPromptDeferred.resolve(attrs)
this.linkPromptDeferred = null
}
}
},
beforeDestroy () {
if (this.quill) {
this.quill.destroy()
this.quill = null
}
},
methods: {
showLinkPrompt () {
// 创建一个 Deferred 对象,用于等待用户输入链接地址
this.linkPromptDeferred = new Deferred()
// 弹出提示框
const url = prompt('请输入链接地址:')
// 如果用户输入了链接地址,则保存链接
if (url) {
this.linkPromptDeferred.resolve({ href: url })
this.linkPromptDeferred = null
}
// 返回 Deferred 对象的 Promise
return this.linkPromptDeferred.promise
}
}
}
</script>
```
相关推荐
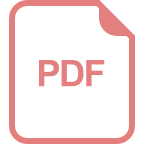
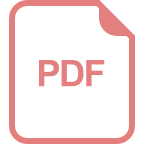














