使用Idea JAva Workbook读取指定路径的Excel,并从指定行开始写入数据
时间: 2024-04-17 14:25:01 浏览: 22
您可以使用 Apache POI 库来读取和写入 Excel 文件。以下是一个使用 Apache POI 的示例代码,它使用 HSSFWorkbook 类来读取和写入 Excel:
```java
org.apache.poi.hssf.usermodel.HSSFWorkbook;
import org.apache.poi.ss.usermodel.*;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
public class ExcelUtils {
public static void readExcel(String filePath, int startRow) {
try {
FileInputStream fis = new FileInputStream(filePath);
Workbook workbook = new HSSFWorkbook(fis);
Sheet sheet = workbook.getSheetAt(0);
for (int i = startRow; i <= sheet.getLastRowNum(); i++) {
Row row = sheet.getRow(i);
Cell cell = row.getCell(0); // Assuming you want to read data from the first column
System.out.println(cell.getStringCellValue());
}
fis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
public static void writeExcel(String filePath, int startRow, String data) {
try {
FileInputStream fis = new FileInputStream(filePath);
Workbook workbook = new HSSFWorkbook(fis);
Sheet sheet = workbook.getSheetAt(0);
Row row = sheet.getRow(startRow);
Cell cell = row.createCell(0); // Assuming you want to write data to the first column
cell.setCellValue(data);
FileOutputStream fos = new FileOutputStream(filePath);
workbook.write(fos);
fos.close();
fis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
public static void main(String[] args) {
String filePath = "path/to/your/excel/file.xls";
int startRow = 1; // Assuming you want to start reading/writing from the second row
readExcel(filePath, startRow);
writeExcel(filePath, startRow, "New Data");
}
}
```
请确保将 `path/to/your/excel/file.xls` 替换为您实际的 Excel 文件路径。`startRow` 参数表示您要从哪一行开始读取或写入数据。示例代码中使用的是 HSSFWorkbook 类,适用于 `.xls` 格式的 Excel 文件。如果您使用的是 `.xlsx` 格式的 Excel 文件,可以使用 XSSFWorkbook 类。
希望这可以帮助到您!
相关推荐
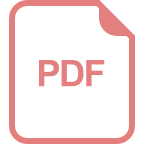
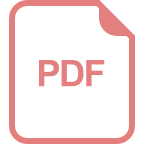
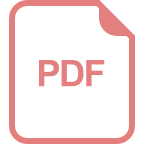













