在编程实战中,如何选择合适的排序算法以达到最优的时间复杂度,并给出相应的代码实现?
时间: 2024-11-11 16:32:02 浏览: 30
选择一个高效的排序算法并确保其时间复杂度最优是编程中的一个重要实践。为了帮助你更好地理解和实现这一目标,推荐查看这本《Fundamentals of Algorithmics》。该书不仅介绍了算法的基础知识,还通过详细的例子和证明深入分析了不同算法的特点和适用场景。
参考资源链接:[Fundamentals of Algorithmics](https://wenku.csdn.net/doc/6412b69dbe7fbd1778d475bb?spm=1055.2569.3001.10343)
在编程中实现排序算法时,需要考虑数据的规模、类型、是否已经部分排序以及对时间复杂度和空间复杂度的要求。一个经典的高效排序算法是快速排序(Quicksort),它的平均时间复杂度为O(n log n),非常适合大数据量的排序任务。
快速排序的基本思想是分治策略。选取一个基准元素,然后将数组分为两部分,一部分包含小于基准的元素,另一部分包含大于基准的元素,然后递归地在两个子数组上重复这个过程。
以下是一个快速排序的Python实现示例:
```python
def quicksort(arr):
if len(arr) <= 1:
return arr
pivot = arr[len(arr) // 2]
left = [x for x in arr if x < pivot]
middle = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
return quicksort(left) + middle + quicksort(right)
# 示例数组
arr = [3, 6, 8, 10, 1, 2, 1]
# 调用快速排序函数
sorted_arr = quicksort(arr)
print(sorted_arr)
```
通过这个示例,你能够看到快速排序算法的具体实现。在项目实战中,你需要根据实际情况选择最合适的排序算法。《Fundamentals of Algorithmics》这本书提供了算法按类型分类的深入探讨,帮助你理解每种算法的适用场景和优缺点,从而在实际编程时能够做出更明智的选择。
参考资源链接:[Fundamentals of Algorithmics](https://wenku.csdn.net/doc/6412b69dbe7fbd1778d475bb?spm=1055.2569.3001.10343)
阅读全文
相关推荐












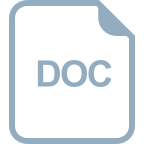





