CBS算法的基本python完整代码
时间: 2024-11-01 09:01:53 浏览: 32
CBS (Conflict-Based Search)算法是一个用于多机器人路径规划的启发式搜索策略,它通过分解任务来管理和处理冲突。以下是基于Python的一个简单版本的CBS算法示例:
```python
from heapq import heappush, heappop
class Node:
def __init__(self, robot, state, cost):
self.robot = robot
self.state = state
self.cost = cost
self.conflicts = []
def __lt__(self, other):
return self.cost < other.cost
class CBS:
def __init__(self, start_states, goal_conditions, conflict_solver):
self.open = []
self.closed = set()
self.start_states = [Node(robot, s, 0) for robot, s in enumerate(start_states)]
self.goal_conditions = goal_conditions
self.conflict_solver = conflict_solver
self.heuristic = lambda n: len(n.state)
def search(self):
while self.open:
node = heappop(self.open)
if self.goal(node):
return self.generate_solution(node)
self.expand(node)
def expand(self, node):
new_nodes = self.generate_children(node)
for child in new_nodes:
if child not in self.closed:
self.closed.add(child)
heappush(self.open, child)
def generate_children(self, parent_node):
children = []
# 解决冲突并添加新状态
for robot, action in self.conflict_solver(parent_node):
child_state = parent_node.state.apply_action(robot, action)
if child_state not in self.closed:
child = Node(robot, child_state, parent_node.cost + 1)
child.conflicts = parent_node.conflicts + [(parent_node.robot, action)]
children.append(child)
return children
def goal(self, node):
return all(self.goal_conditions(robot, node.state) for robot in range(len(node.state)))
def generate_solution(self, node):
solution = [(node.robot, node.state.current_path)]
while node.parent:
node = node.parent
solution.insert(0, (node.robot, node.state.current_path))
return solution
# 使用示例
def conflict_solver(node):
# 在这里实现具体的冲突解决方案...
pass
# 示例开始状态、结束条件等
start_states = [(robot_id, 'start') for robot_id in range(num_robots)]
goal_conditions = lambda r, s: s == 'end'
cbs = CBS(start_states, goal_conditions, conflict_solver)
solution = cbs.search()
阅读全文
相关推荐


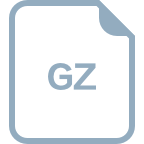







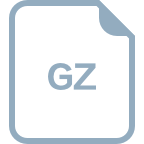

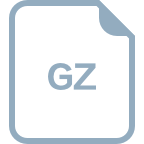

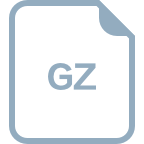
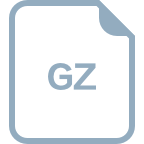


