python实现cbs算法
时间: 2023-09-28 08:12:15 浏览: 365
CBS算法是一种基于冲突搜索的路径规划算法,用于解决多智能体系统的路径规划问题。在Python中实现CBS算法,可以依赖于第三方库例如networkx,numpy等。以下是基本的伪代码实现:
```
//定义状态类,每个状态有当前路径集合和当前冲突集合
class State:
def __init__(self, paths, conflicts):
self.paths = paths
self.conflicts = conflicts
//定义CBS算法的主函数
def cbs(start, goal, constraints):
//初始化起始状态,并将其加入open列表
initial_state = State(path, [])
open_list = [initial_state]
//循环直至找到解或者open列表为空
while open_list:
//从open列表中弹出最小 F 值的状态
current = min(open_list, key=lambda x: x.F)
open_list.remove(current)
//判断当前状态是否是目标状态
if is_goal(current):
return current.paths
//扩展状态并将生成的新状态加入open列表
for state in expand(current, constraints):
open_list.append(state)
return None
//定义状态扩展函数
def expand(state, constraints):
//从当前状态获取所有的冲突
conflicts = state.conflicts
//循环扩展路径并查看是否生成新的冲突
for i in range(len(state.paths)):
for j in range(i+1, len(state.paths)):
if conflict(state.paths[i], state.paths[j], constraints):
//如果存在冲突就生成新路径集合和新冲突集合
new_paths = resolve_conflict(state.paths[i], state.paths[j], conflicts)
new_conflicts = conflicts.append((i, j, t))
//返回新状态
yield State(new_paths, new_conflicts)
//定义相邻路径冲突检测函数
def conflict(path1, path2, constraints):
//定义路径1和路径2之间的交集
intersect = set(path1) & set(path2)
//循环检查每个时间步骤是否符合约束条件
for t in intersect:
for c in constraints:
if c.check(path1, path2, t):
//如果有任一时间步骤违反了约束条件,则判断为冲突
return True
//如果没有冲突返回False
return False
//定义解决冲突函数
def resolve_conflict(path1, path2, conflicts):
//找到所有冲突涉及到的时间步骤
timesteps = set()
for c in conflicts:
if c[0] == path1 or c[1] == path1 or c[0] == path2 or c[1] == path2:
timesteps.add(c[2])
//将所有与路径1和路径2相关的时间步骤合并成一个新路径
new_path = []
for t in range(max([max(path1), max(path2)], default=0)):
if t in timesteps:
new_path.append(path2[t])
else:
new_path.append(path1[t])
//返回合并后的新路径
return new_path
```
阅读全文
相关推荐




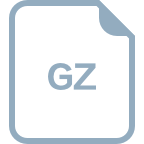








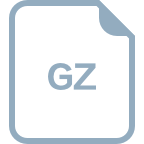

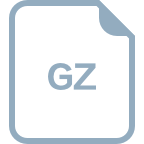
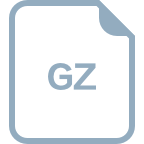

