python编写代码实现:模拟游戏“砸金花”,生成扑克牌,判断一对、三条、一顺、同花顺、同花、普通出现的概率,可以模拟发1万次牌,对出现的次数进行统计
时间: 2024-09-30 20:09:03 浏览: 27
在Python中,我们可以利用random库来模拟砸金花游戏,其中包含洗牌、发牌以及判断各种牌型概率的功能。首先,我们需要定义一副完整的扑克牌,然后实现相应的函数来处理各种牌型判断。以下是一个简化的示例:
```python
import random
# 定义扑克牌及其概率
suits = ['♠', '♥', '♦', '♣']
ranks = ['A', '2', '3', '4', '5', '6', '7', '8', '9', '10', 'J', 'Q', 'K']
deck = [(rank, suit) for rank in ranks for suit in suits]
random.shuffle(deck) # 洗牌
def deal_card():
return deck.pop()
def count_pairs(hand):
pairs = [card for card in hand if hand.count(card[0]) == 2]
return len(pairs)
def count_threes(hand):
threes = [card for card in hand if hand.count(card[0]) == 3]
return len(threes)
def count_straight(hand):
straight_ranks = sorted([int(rank) for rank in hand])
is_straight = all(straight_ranks[i] - straight_ranks[i - 1] == 1 for i in range(1, len(straight_ranks)))
return int(is_straight)
def check_flush(hand):
suit_count = {suit: hand.count(suit) for suit in suits}
return max(suit_count.values()) >= 5
def check_straight_flush(hand):
sorted_hand = sorted(hand)
is_straight = sorted_hand[:-1] == list(range(sorted_hand[0][0], sorted_hand[-1][0]+1))
return is_straight and check_flush(hand)
def simulate_game(num_trials=10000):
result_counts = {
'Pairs': 0,
'Threes': 0,
'Straight': 0,
'Flush': 0,
'Straight Flush': 0
}
for _ in range(num_trials):
hand = [deal_card() for _ in range(5)]
pairs = count_pairs(hand)
threes = count_threes(hand)
straight = count_straight(hand)
flush = check_flush(hand)
straight_flush = check_straight_flush(hand)
result_counts['Pairs'] += pairs
result_counts['Threes'] += threes
result_counts['Straight'] += straight
result_counts['Flush'] += flush
result_counts['Straight Flush'] += straight_flush
probabilities = {k: v / num_trials for k, v in result_counts.items()}
return probabilities, result_counts
# 测试并统计结果
probabilities, counts = simulate_game(10000)
print(f"Probability distribution after {num_trials} trials:")
for key, value in probabilities.items():
print(f"{key}: {value * 100:.2f}% (count: {counts[key]})")
```
这个脚本会模拟砸金花游戏中发牌1万次,并计算各种牌型出现的频率。运行后,你会看到每种牌型出现的概率以及各自的出现次数。
阅读全文
相关推荐
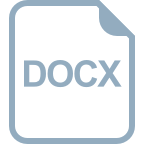
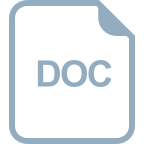
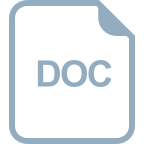

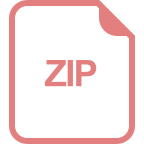
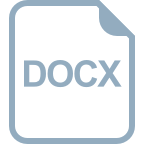
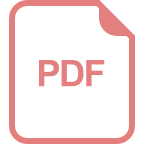
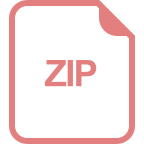
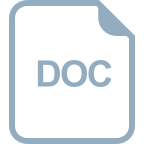
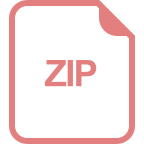
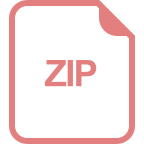
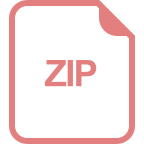
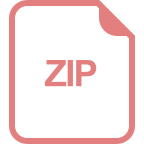