random python some code
时间: 2024-12-22 08:11:13 浏览: 4
Python的`random`模块提供了一系列强大的功能来生成随机数。这里有一些基本的用法示例[^1]:
1. **生成随机浮点数**:
```python
from random import uniform
random_float = uniform(0, 1) # 生成0到1之间的随机浮点数
```
2. **生成随机整数**:
```python
from random import randint
random_int = randint(1, 100) # 生成1到100之间的随机整数
```
3. **从列表中随机选择元素**:
```python
numbers = [1, 2, 3, 4, 5]
chosen_num = random.choice(numbers)
```
4. **打乱列表顺序**(例如,洗牌):
```python
shuffled_list = random.sample(numbers, len(numbers))
```
5. **随机种子设置**(为了可重复性):
```python
random.seed(42) # 设置固定种子以获得相同的结果
```
Java中也有相似的功能,但这里我们专注于Python。如果你想了解Java版本的随机数生成,可以查看相关文档。
相关问题
Python code of WLS
WLS (Weighted Least Squares) is a method used to estimate the parameters of a linear regression model when the errors are heteroscedastic (i.e., the variance of the errors is not constant). Here is an example Python code of WLS:
```python
import numpy as np
import statsmodels.api as sm
# Generate some data
x = np.arange(10)
y = 2*x + 5 + np.random.normal(0, 1, 10) # Heteroscedastic errors
# Fit a linear regression model using WLS
weights = 1 / np.arange(1, 11) # Weights are inversely proportional to x
X = sm.add_constant(x)
model = sm.WLS(y, X, weights=weights)
results = model.fit()
# Print the results
print(results.summary())
```
In this example, we generate some data with heteroscedastic errors and fit a linear regression model using WLS. The weights are chosen to be inversely proportional to x (i.e., higher weights are assigned to smaller values of x), which is a common choice in practice. The `sm.WLS` function from the `statsmodels` library is used to perform the WLS estimation, and the results are printed using the `summary` method of the `results` object.
fick diffusion code using random walk method
Sure, I can help you with that. The random walk method is commonly used to simulate diffusion processes in materials. Here's some sample code in Python that implements the random walk method:
```python
import numpy as np
import matplotlib.pyplot as plt
# Define simulation parameters
N = 1000 # Number of particles
steps = 1000 # Number of steps
L = 100 # Size of the system
D = 1 # Diffusion constant
# Initialize particle positions
x = np.zeros(N)
y = np.zeros(N)
# Run simulation
for i in range(steps):
# Update particle positions
x += np.sqrt(2*D)*np.random.randn(N)
y += np.sqrt(2*D)*np.random.randn(N)
# Reflect particles at system boundaries
x = np.mod(x, L)
y = np.mod(y, L)
# Plot particle positions
if i % 10 == 0:
plt.clf()
plt.plot(x, y, 'bo')
plt.xlim([0, L])
plt.ylim([0, L])
plt.draw()
plt.pause(0.0001)
```
In this code, we define the simulation parameters such as the number of particles `N`, the number of steps `steps`, the size of the system `L`, and the diffusion constant `D`. We then initialize the particle positions to be at the origin.
We then loop over the number of steps and update the particle positions using the random walk method. We also reflect the particles at the system boundaries to ensure they stay within the system.
Finally, we plot the particle positions at every 10th step using matplotlib. The `plt.pause(0.0001)` function is used to update the plot in real-time.
Hope this helps! Let me know if you have any questions.
阅读全文
相关推荐
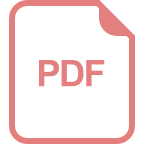
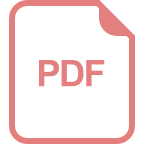
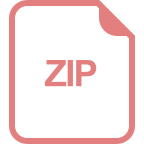













