#include <stdio.h> #include <stdlib.h> #include <string.h> #define MAX_LENGTH 100 typedef struct { char word[MAX_LENGTH]; int count; } WordCount; int main() { char searchWord[MAX_LENGTH]; int i, j, found; WordCount *words = NULL; int wordsCount = 0; // 打开文件 FILE *file = fopen("ss.txt", "r"); // 读取文件内容,逐个单词进行统计 char word[MAX_LENGTH]; while (fscanf(file, "%s", word) != EOF) { found = 0; // 检查该单词是否已经存在于统计数组中 for (i = 0; i < wordsCount; i++) { if (strcmp(word, words[i].word) == 0) { found = 1; words[i].count++; break; } } // 如果单词不存在于统计数组中,则将其添加到数组中 if (!found) { words = (WordCount*)realloc(words, (wordsCount + 1) * sizeof(WordCount)); strcpy(words[wordsCount].word, word); words[wordsCount].count = 1; wordsCount++; } } // 关闭文件 fclose(file); // 输入要查找的单词 printf("请输入要查找的单词:"); scanf("%s", searchWord); // 在统计数组中查找该单词并输出重复次数 found = 0; for (i = 0; i < wordsCount; i++) { if (strcmp(searchWord, words[i].word) == 0) { printf("单词 %s 出现了 %d 次\n", searchWord, words[i].count); found = 1; break; } } // 如果未找到该单词,则输出未找到的提示信息 if (!found) { printf("未找到单词 %s\n", searchWord); } // 释放内存 free(words); return 0; }逐行解释代码
时间: 2024-02-14 14:26:02 浏览: 69
好的,以下是对代码逐行的解释:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LENGTH 100
typedef struct {
char word[MAX_LENGTH];
int count;
} WordCount;
int main() {
char searchWord[MAX_LENGTH];
int i, j, found;
WordCount *words = NULL;
int wordsCount = 0;
```
这部分是包含头文件、定义常量、定义结构体以及声明变量的部分。
```c
// 打开文件
FILE *file = fopen("ss.txt", "r");
```
这行代码打开名为 "ss.txt" 的文件,以只读方式打开。
```c
// 读取文件内容,逐个单词进行统计
char word[MAX_LENGTH];
while (fscanf(file, "%s", word) != EOF) {
found = 0;
// 检查该单词是否已经存在于统计数组中
for (i = 0; i < wordsCount; i++) {
if (strcmp(word, words[i].word) == 0) {
found = 1;
words[i].count++;
break;
}
}
// 如果单词不存在于统计数组中,则将其添加到数组中
if (!found) {
words = (WordCount*)realloc(words, (wordsCount + 1) * sizeof(WordCount));
strcpy(words[wordsCount].word, word);
words[wordsCount].count = 1;
wordsCount++;
}
}
```
这部分代码逐个单词读取文件内容,并进行统计。使用 `fscanf` 函数从文件中读取单词,如果读取到文件末尾(EOF),则停止循环。在循环中,检查该单词是否已经存在于统计数组中,如果存在则增加计数,如果不存在则将其添加到数组中。
```c
// 关闭文件
fclose(file);
```
这行代码关闭文件。
```c
// 输入要查找的单词
printf("请输入要查找的单词:");
scanf("%s", searchWord);
```
这部分代码用于输入要查找的单词。
```c
// 在统计数组中查找该单词并输出重复次数
found = 0;
for (i = 0; i < wordsCount; i++) {
if (strcmp(searchWord, words[i].word) == 0) {
printf("单词 %s 出现了 %d 次\n", searchWord, words[i].count);
found = 1;
break;
}
}
```
这部分代码在统计数组中查找输入的单词,并输出其重复次数。
```c
// 如果未找到该单词,则输出未找到的提示信息
if (!found) {
printf("未找到单词 %s\n", searchWord);
}
```
这部分代码用于处理未找到单词的情况,输出未找到的提示信息。
```c
// 释放内存
free(words);
return 0;
}
```
最后,这行代码用于释放动态分配的内存,并返回程序执行成功的标志。
希望这样的解释对你有帮助!如果还有其他问题,请随时提问。
阅读全文
相关推荐
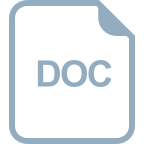
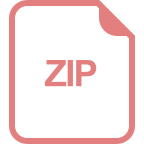















