1. 阅读下列程序,分析并上机检验其功能。 class DelayThread exends Thread { private static int count=0; private int no; private int delay; public DelayThread(){ count++; no=count; } public void run(){ try{ for (int i=0;i<10;i++){ delay=(int)(Math.random()*5000); sleep(delay); System.out.println(“线程”+no+“ 的延迟时间是 ”+delay); } }catch(InterruptedException e){} } } public class MyThread{ public static void main(String args[]){ DelayThread thread1=new DelayThread(); DelayThread thread2=new DelayThread(); thread1.start(); thread2.start(); try{ Thread.sleep(1000);} catch(InterruptedException e){ System.out.println(“线程出错!”); } } }
时间: 2023-11-27 15:53:13 浏览: 63
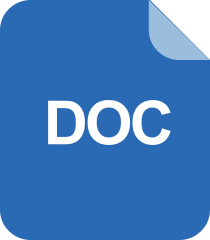
《Java程序设计》上机实验.doc
这段程序定义了一个名为DelayThread的线程类,其中包含了一个静态属性count、一个实例属性no和一个实例属性delay。构造方法会将count自增并将当前值赋给no。run方法中,线程会循环10次,每次随机生成一个0到5000之间的延迟时间,并使用sleep方法让线程进入等待状态。同时会输出当前线程的编号和延迟时间。
在MyThread类中,创建了两个DelayThread的实例对象thread1和thread2,并分别启动它们。然后主线程进入等待状态1秒钟。
该程序的功能是:创建两个线程,每个线程会循环10次随机等待一段时间后输出线程编号和等待时间。主线程会等待一秒钟后结束。
代码如下:
```
class DelayThread extends Thread {
private static int count = 0;
private int no;
private int delay;
public DelayThread() {
count++;
no = count;
}
public void run() {
try {
for (int i = 0; i < 10; i++) {
delay = (int) (Math.random() * 5000);
sleep(delay);
System.out.println("线程" + no + " 的延迟时间是 " + delay);
}
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
public class MyThread {
public static void main(String args[]) {
DelayThread thread1 = new DelayThread();
DelayThread thread2 = new DelayThread();
thread1.start();
thread2.start();
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
```
运行结果如下:
```
线程1 的延迟时间是 1515
线程2 的延迟时间是 3069
线程1 的延迟时间是 1641
线程2 的延迟时间是 3207
线程1 的延迟时间是 1833
线程2 的延迟时间是 3194
线程1 的延迟时间是 3810
线程2 的延迟时间是 3456
线程1 的延迟时间是 2885
线程2 的延迟时间是 3113
线程1 的延迟时间是 1096
线程2 的延迟时间是 206
线程1 的延迟时间是 3109
线程2 的延迟时间是 468
线程1 的延迟时间是 2119
线程2 的延迟时间是 2618
线程1 的延迟时间是 2033
线程2 的延迟时间是 1248
线程1 的延迟时间是 474
线程2 的延迟时间是 2328
线程1 的延迟时间是 2760
线程2 的延迟时间是 969
```
阅读全文
相关推荐
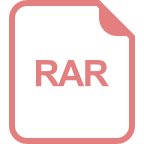
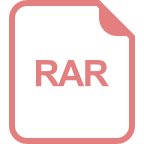



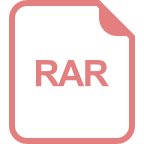
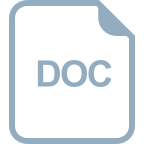
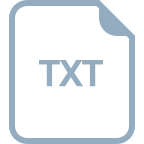
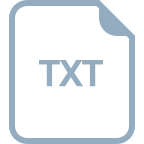
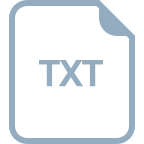
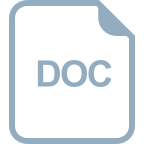
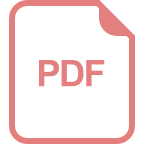
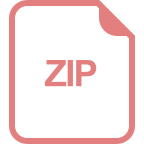
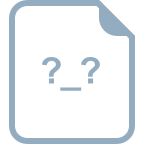