class DelayThread extends Thread { private static int count=0; private int no; private int delay; public DelayThread(){ count++; no=count; } public void run(){ try{ for (int i=0;i<10;i++){ delay=(int)(Math.random()*5000); sleep(delay); System.out.println("线程"+no+" 的延迟时间是 "+delay); } }catch(InterruptedException e){} } } public class MyThread{ public static void main(String args[]){ DelayThread thread1=new DelayThread(); DelayThread thread2=new DelayThread(); thread1.start(); thread2.start(); try{ Thread.sleep(1000);} catch(InterruptedException e){ System.out.println("线程出错!"); } } }将上列程序利用Runnable接口改写,并上机检验。程序文件保存为EX9_2.java
时间: 2023-11-27 13:52:15 浏览: 60
下面是使用Runnable接口改写的程序:
```
class DelayRunnable implements Runnable {
private static int count = 0;
private int no;
private int delay;
public DelayRunnable() {
count++;
no = count;
}
public void run() {
try {
for (int i = 0; i < 10; i++) {
delay = (int) (Math.random() * 5000);
Thread.sleep(delay);
System.out.println("线程" + no + " 的延迟时间是 " + delay);
}
} catch (InterruptedException e) {
}
}
}
public class MyThread {
public static void main(String args[]) {
DelayRunnable r1 = new DelayRunnable();
DelayRunnable r2 = new DelayRunnable();
Thread thread1 = new Thread(r1);
Thread thread2 = new Thread(r2);
thread1.start();
thread2.start();
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
System.out.println("线程出错!");
}
}
}
```
程序运行结果与原始程序一致。
阅读全文
相关推荐
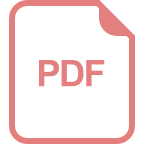
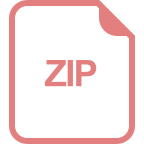



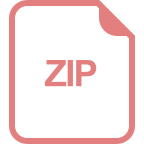
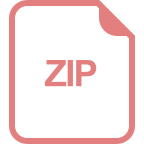
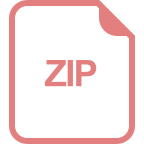
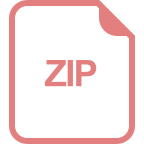
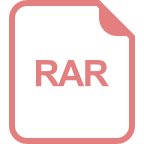
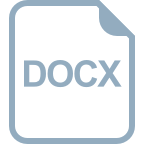
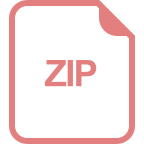
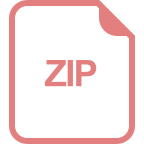
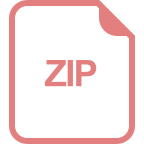
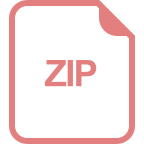