点击 iframe 时在 window 上无法监听到 click 事件,关闭不了页面
时间: 2024-02-27 08:59:26 浏览: 150
这可能是因为 iframe 和 window 属于不同的文档,因此 window 上的事件无法直接监听到 iframe 上的事件。解决这个问题的一种方法是,在 iframe 内部添加一个监听器,并且在监听器中使用 `window.parent` 来调用父窗口中的函数来关闭页面。例如,可以在 iframe 中添加以下代码:
```javascript
document.addEventListener('click', function() {
window.parent.closePage();
});
```
然后在父窗口中添加 `closePage()` 函数来关闭页面。这样,当用户在 iframe 中点击时,就会触发 iframe 中的监听器,然后调用父窗口中的函数来关闭页面。
相关问题
监听嵌套在vue中iframe页面中的按钮的点击事件,在点按钮点击时,先处理自己的逻辑,再执行iframe的逻辑,并且不知道iframe页面的方法和按钮是没有固定id和class
可以通过以下步骤实现:
1. 获取到iframe元素,可以使用`document.querySelector()`或`document.getElementById()`方法获取到。
2. 使用`iframe.contentWindow`获取到iframe的window对象,进而获取到iframe页面中的document对象。
3. 使用`document.querySelectorAll()`方法获取所有的按钮元素,然后遍历它们,为它们添加点击事件处理函数。
4. 在点击事件处理函数中,先处理自己的逻辑,然后再调用iframe页面中的方法。
以下是示例代码:
```
// 获取iframe元素
const iframe = document.querySelector('iframe')
// 获取iframe页面中的document对象
const iframeDoc = iframe.contentWindow.document
// 获取所有按钮元素并为它们添加点击事件处理函数
const buttons = iframeDoc.querySelectorAll('button')
buttons.forEach(button => {
button.addEventListener('click', () => {
// 处理自己的逻辑
console.log('Button clicked')
// 调用iframe页面中的方法
iframeDoc.someFunction()
})
})
```
需要注意的是,由于iframe中的页面可能涉及到跨域问题,因此需要确保父页面和iframe页面都在同一个域名下,否则可能会受到同源策略的限制。
iframe 编辑内部页面的输入框及事件监听
可以通过以下步骤实现:
1. 在父页面中添加一个 iframe 标签,设置其 src 属性为要嵌入的页面的 URL。
2. 在 iframe 中的页面中添加需要监听的事件。
3. 在父页面中获取 iframe 中的输入框元素,以及其他需要监听的元素。
4. 使用 JavaScript 中的 postMessage() 方法向 iframe 中发送消息,监听 iframe 中的事件并做出相应处理。
下面是一个示例代码:
父页面代码:
```html
<!DOCTYPE html>
<html>
<head>
<title>父页面</title>
</head>
<body>
<iframe id="my-iframe" src="inner-page.html"></iframe>
<script>
// 获取 iframe 中的输入框元素
var iframe = document.getElementById("my-iframe");
var innerInput = iframe.contentWindow.document.getElementById("inner-input");
// 监听 iframe 中的事件
window.addEventListener("message", function(event) {
if (event.origin !== "http://localhost:8000") {
return;
}
// 处理事件
if (event.data.type === "input") {
console.log("输入框的值为:" + event.data.value);
} else if (event.data.type === "button") {
console.log("点击了按钮!");
}
});
// 向 iframe 发送消息
function sendMessage(type, value) {
iframe.contentWindow.postMessage({
type: type,
value: value
}, "*");
}
</script>
</body>
</html>
```
内部页面代码:
```html
<!DOCTYPE html>
<html>
<head>
<title>内部页面</title>
</head>
<body>
<input type="text" id="inner-input">
<button id="inner-button">点击我</button>
<script>
// 监听输入框的输入事件
var innerInput = document.getElementById("inner-input");
innerInput.addEventListener("input", function() {
// 向父页面发送消息
window.parent.postMessage({
type: "input",
value: innerInput.value
}, "*");
});
// 监听按钮的点击事件
var innerButton = document.getElementById("inner-button");
innerButton.addEventListener("click", function() {
// 向父页面发送消息
window.parent.postMessage({
type: "button"
}, "*");
});
</script>
</body>
</html>
```
在这个示例中,父页面和内部页面都是同一个域名下的页面,所以使用了通配符 "*" 来接受所有来源的消息。在实际应用中,应该根据需要设置特定的来源。
阅读全文
相关推荐
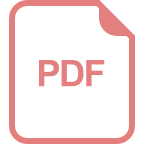
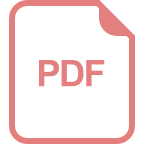
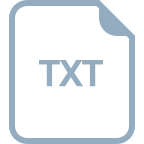








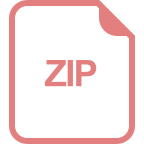




