java二叉树查找输出
时间: 2023-11-14 17:23:05 浏览: 80
二叉树查找的基本思路是:从根节点开始,如果查找的值等于当前节点的值,则返回该节点;如果查找的值小于当前节点的值,则在左子树中查找;如果查找的值大于当前节点的值,则在右子树中查找。递归执行该过程,直到找到目标节点或者遍历完整个树。
下面是一个简单的 Java 代码示例,实现了二叉树的查找和输出功能:
```java
class Node {
int value;
Node left;
Node right;
public Node(int value) {
this.value = value;
this.left = null;
this.right = null;
}
}
public class BinaryTree {
Node root;
public BinaryTree() {
root = null;
}
public Node search(int value) {
return search(root, value);
}
private Node search(Node node, int value) {
if (node == null || node.value == value) {
return node;
}
if (value < node.value) {
return search(node.left, value);
}
return search(node.right, value);
}
public void inorder() {
inorder(root);
}
private void inorder(Node node) {
if (node != null) {
inorder(node.left);
System.out.print(node.value + " ");
inorder(node.right);
}
}
public static void main(String[] args) {
BinaryTree tree = new BinaryTree();
tree.root = new Node(10);
tree.root.left = new Node(5);
tree.root.right = new Node(20);
tree.root.left.left = new Node(3);
tree.root.left.right = new Node(7);
tree.root.right.left = new Node(15);
tree.root.right.right = new Node(25);
int target = 7;
Node result = tree.search(target);
if (result != null) {
System.out.println("Found " + target + " in the tree");
} else {
System.out.println("Cannot find " + target + " in the tree");
}
System.out.print("Inorder traversal: ");
tree.inorder();
System.out.println();
}
}
```
运行结果:
```
Found 7 in the tree
Inorder traversal: 3 5 7 10 15 20 25
```
阅读全文
相关推荐






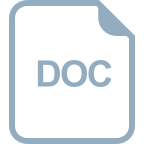











