c++ 读取word文档内容
时间: 2023-08-04 18:38:23 浏览: 412
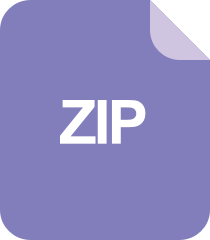
VC 读取word文档内容_VC60_word
要在C++中读取Word文档内容,可以使用Microsoft Office自带的COM接口,通过COM接口调用Word应用程序的API来实现。具体步骤如下:
1. 引用Word应用程序库文件
在Visual Studio中,可以通过添加引用来引用Word应用程序库文件。在Solution Explorer中右键点击项目名称,选择"Add Reference",在COM中找到"Microsoft Word xx.x Object Library",选择后点击"Add"添加引用。
2. 创建Word应用程序对象
通过COM接口创建Word应用程序对象,并打开指定的Word文档。
```
#include <windows.h>
#include <ole2.h>
#include <oleauto.h>
#include <atlbase.h>
#include <atlcom.h>
#include <atlctl.h>
#include <atlwin.h>
#include <atlstr.h>
#include <comutil.h>
#include <msword.olb>
using namespace Word;
_ApplicationPtr pApp; // Word应用程序对象指针
DocumentsPtr pDocs; // Word文档对象指针
_DocumentPtr pDoc; // 操作的Word文档对象指针
// 初始化COM库
HRESULT hr = CoInitialize(NULL);
// 创建Word应用程序对象
hr = pApp.CreateInstance(__uuidof(Application));
// 打开Word文档
hr = pApp->Documents->Open(_bstr_t("C:\\test.docx"), vtMissing, vtMissing, vtMissing, vtMissing, vtMissing, vtMissing, vtMissing, vtMissing, vtMissing, vtMissing, vtMissing, vtMissing, vtMissing, vtMissing, vtMissing);
```
3. 读取Word文档内容
通过Word文档对象的API读取文档内容,例如,可以获取文档的段落、表格、图片等信息。
```
// 获取文档段落数
long paraCount = pDoc->Paragraphs->Count;
// 读取文档某个段落的文本内容
ParagraphPtr pPara = pDoc->Paragraphs->Item(1);
_bstr_t paraText = pPara->Range->Text;
// 获取文档表格数
long tableCount = pDoc->Tables->Count;
// 读取文档某个表格的内容
TablePtr pTable = pDoc->Tables->Item(1);
int rowCount = pTable->Rows->Count;
int colCount = pTable->Columns->Count;
for (int i = 1; i <= rowCount; i++) {
for (int j = 1; j <= colCount; j++) {
CellPtr pCell = pTable->Cell(i, j);
_bstr_t cellText = pCell->Range->Text;
}
}
// 获取文档图片数
long shapeCount = pDoc->Shapes->Count;
for (int i = 1; i <= shapeCount; i++) {
ShapePtr pShape = pDoc->Shapes->Item(i);
if (pShape->Type == msoPicture) {
// 处理图片
}
}
```
4. 释放资源
完成操作后,需要及时释放资源。
```
// 关闭文档
pDoc->Close(vtMissing, vtMissing, vtMissing);
// 退出Word应用程序
pApp->Quit();
// 释放COM对象
pDoc.Release();
pDocs.Release();
pApp.Release();
CoUninitialize();
```
阅读全文
相关推荐
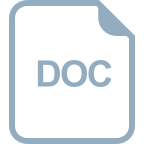
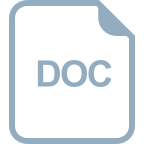



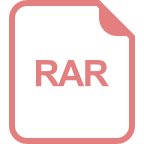
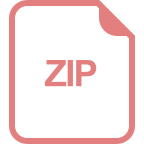
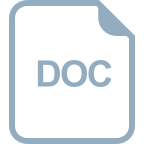
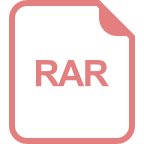
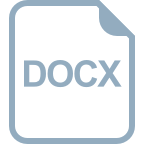
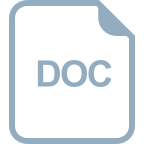
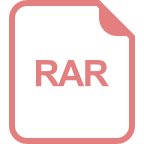





