使用C++ 读取word文档
时间: 2023-09-26 20:09:34 浏览: 222
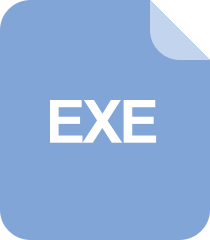
关于C++的word文档
要使用C++读取Word文档,一种常用的方法是使用Microsoft Office提供的COM接口。以下是一个简单的示例代码,可以读取Word文档中的文本内容:
```c++
#include <windows.h>
#include <iostream>
#include <string>
#include <vector>
// Microsoft Office COM interface
#import "C:\\Program Files\\Common Files\\Microsoft Shared\\OFFICE16\\MSO.DLL" \
rename("DocumentProperties", "OfficeDocumentProperties") \
rename("RGB", "MSORGB") \
rename("DialogBox", "MSODialogBox") \
rename("CopyFile", "OfficeCopyFile")
#import "C:\\Program Files\\Microsoft Office\\Root\\Office16\\WORD.EXE" \
rename("ExitWindows", "WordExitWindows") \
rename("FindText", "WordFindText") \
rename("ReplaceText", "WordReplaceText") \
rename("Selection", "WordSelection") \
rename("Font", "WordFont") \
rename("ParagraphFormat", "WordParagraphFormat") \
rename("TextRetrievalMode", "WordTextRetrievalMode") \
exclude("OLE_HANDLE", "OLE_COLOR", "OLE_OPTEXCLUSIVE", "OLE_CANCELBOOL", "OLE_XPOS_PIXELS", "OLE_YPOS_PIXELS", "OLE_XSIZE_PIXELS", "OLE_YSIZE_PIXELS", "OLE_XPOS_CONTAINER", "OLE_YPOS_CONTAINER", "OLE_XSIZE_CONTAINER", "OLE_YSIZE_CONTAINER", "OLE_HANDLE", "OLE_HANDLE_PTR_TYPE")
using namespace std;
int main()
{
CoInitialize(NULL);
try {
// Create an instance of the Word application
_ApplicationPtr Word("Word.Application");
Word->Visible = VARIANT_FALSE;
// Open the Word document
_DocumentPtr doc = Word->Documents->Open("path/to/your/word/document.docx");
// Read text content
vector<string> content;
for (Paragraph paragraph : doc->Paragraphs) {
string text = paragraph.Range->Text;
content.push_back(text);
}
// Print text content
for (string line : content) {
cout << line << endl;
}
// Close the document and quit the Word application
doc->Close(VARIANT_FALSE);
Word->Quit(VARIANT_FALSE);
}
catch (_com_error& e) {
cout << "Error: " << e.ErrorMessage() << endl;
}
CoUninitialize();
return 0;
}
```
此代码使用COM接口创建了一个Word应用程序实例,并打开指定路径下的Word文档。然后,它遍历文档的每个段落并读取其中的文本内容。最后,它输出了读取到的文本内容,并关闭了文档和Word应用程序。
请注意,这段代码需要安装Microsoft Office套件并引入相应的COM接口。
阅读全文
相关推荐


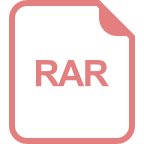
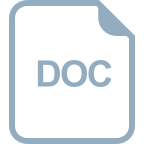




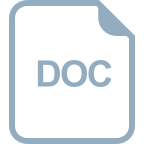
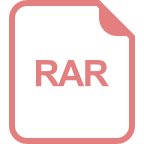
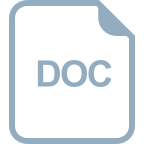
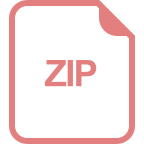
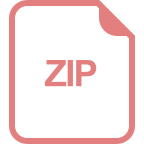
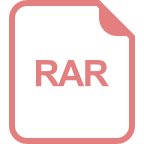
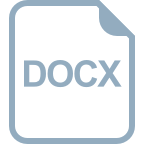
