在页面上实现一个可以预览的9宫格图片,然后再把图片上传到后台的服务器硬盘上
时间: 2024-05-09 19:14:21 浏览: 102
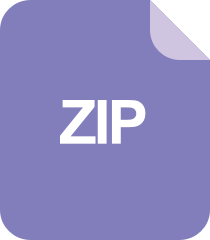
Android平台仿微信朋友圈的9宫格图片展示与多选功能教程
首先,我们可以使用 HTML 和 CSS 来创建一个简单的 9 宫格图片预览。代码如下:
```html
<!DOCTYPE html>
<html>
<head>
<title>9宫格图片上传</title>
<style>
.container {
display: flex;
flex-wrap: wrap;
justify-content: center;
align-items: center;
}
.image {
width: 30%;
margin: 10px;
border: 1px solid #ccc;
text-align: center;
padding: 5px;
box-sizing: border-box;
}
.image img {
max-width: 100%;
max-height: 100%;
}
.file-input {
display: none;
}
.file-label {
background-color: #3498db;
color: #fff;
padding: 10px 20px;
border-radius: 5px;
text-align: center;
cursor: pointer;
transition: all 0.3s ease;
}
.file-label:hover {
background-color: #2980b9;
}
</style>
</head>
<body>
<div class="container">
<div class="image">
<label for="file-input-1">
<img src="https://via.placeholder.com/150x150" alt="Placeholder">
</label>
<input type="file" class="file-input" id="file-input-1">
</div>
<div class="image">
<label for="file-input-2">
<img src="https://via.placeholder.com/150x150" alt="Placeholder">
</label>
<input type="file" class="file-input" id="file-input-2">
</div>
<div class="image">
<label for="file-input-3">
<img src="https://via.placeholder.com/150x150" alt="Placeholder">
</label>
<input type="file" class="file-input" id="file-input-3">
</div>
<div class="image">
<label for="file-input-4">
<img src="https://via.placeholder.com/150x150" alt="Placeholder">
</label>
<input type="file" class="file-input" id="file-input-4">
</div>
<div class="image">
<label for="file-input-5">
<img src="https://via.placeholder.com/150x150" alt="Placeholder">
</label>
<input type="file" class="file-input" id="file-input-5">
</div>
<div class="image">
<label for="file-input-6">
<img src="https://via.placeholder.com/150x150" alt="Placeholder">
</label>
<input type="file" class="file-input" id="file-input-6">
</div>
<div class="image">
<label for="file-input-7">
<img src="https://via.placeholder.com/150x150" alt="Placeholder">
</label>
<input type="file" class="file-input" id="file-input-7">
</div>
<div class="image">
<label for="file-input-8">
<img src="https://via.placeholder.com/150x150" alt="Placeholder">
</label>
<input type="file" class="file-input" id="file-input-8">
</div>
<div class="image">
<label for="file-input-9">
<img src="https://via.placeholder.com/150x150" alt="Placeholder">
</label>
<input type="file" class="file-input" id="file-input-9">
</div>
</div>
<script>
const fileInputs = document.querySelectorAll('.file-input');
fileInputs.forEach(fileInput => {
fileInput.addEventListener('change', event => {
const file = event.target.files[0];
const reader = new FileReader();
reader.readAsDataURL(file);
reader.onload = event => {
const image = event.target.result;
const imageElement = fileInput.previousElementSibling;
imageElement.src = image;
};
});
});
</script>
</body>
</html>
```
以上代码中,我们使用了 Flexbox 布局来创建 9 个等宽的图片容器。每个容器包含一个 label 元素和一个 input[type="file"] 元素。label 元素中包含一个 img 元素,用于显示预览图片。input[type="file"] 元素被隐藏,但是当用户点击对应的 label 元素时,就会触发 input[type="file"] 元素的点击事件,从而打开文件上传对话框。
接下来,我们需要使用 JavaScript 来实现将上传的图片保存到服务器上。由于这需要使用服务器端编程语言(如 PHP、Python 等),所以这里我们只提供一个简单的示例代码,演示如何将图片数据通过 AJAX 发送给服务器。这个示例代码假设服务器端的上传接口是 `/upload`,上传的图片数据被保存在名为 `image` 的 POST 参数中。
```javascript
const fileInputs = document.querySelectorAll('.file-input');
fileInputs.forEach(fileInput => {
fileInput.addEventListener('change', event => {
const file = event.target.files[0];
const reader = new FileReader();
reader.readAsDataURL(file);
reader.onload = event => {
const image = event.target.result;
const xhr = new XMLHttpRequest();
xhr.open('POST', '/upload');
xhr.setRequestHeader('Content-Type', 'application/x-www-form-urlencoded');
xhr.onreadystatechange = () => {
if (xhr.readyState === 4 && xhr.status === 200) {
console.log('Image uploaded successfully');
}
};
xhr.send(`image=${encodeURIComponent(image)}`);
};
});
});
```
以上代码中,我们为每个 input[type="file"] 元素添加了一个 change 事件监听器。当用户选择文件后,会触发该监听器,从而创建一个 FileReader 对象来读取文件数据。读取完成后,我们使用 XMLHttpRequest 对象将图片数据发送给服务器。服务器可以根据需要将图片数据保存到磁盘上。需要注意的是,这个示例代码中的上传接口是不安全的,因为它可以接受任何数据,包括恶意代码。实际应用中,我们需要在服务器端对上传的数据进行检查和过滤,以确保安全性。
阅读全文
相关推荐
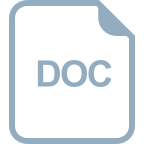
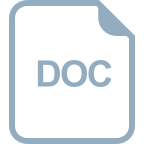
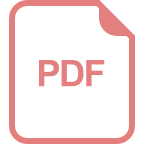
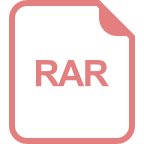
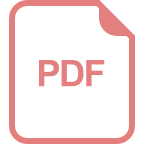
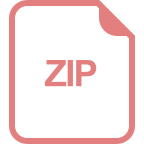

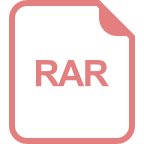
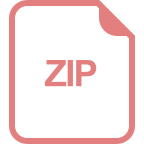
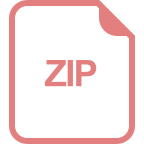
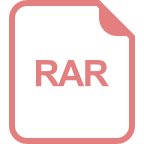



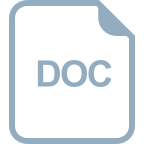
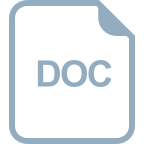