如何接入apollo,实现spring依赖注入的bean动态刷新
时间: 2023-07-20 13:18:47 浏览: 149
接入Apollo实现Spring依赖注入的Bean动态刷新,需要以下步骤:
1. 引入Apollo的依赖
在Maven或Gradle中添加Apollo的依赖:
Maven:
```xml
<dependency>
<groupId>com.ctrip.framework.apollo</groupId>
<artifactId>apollo-client</artifactId>
<version>${apollo.version}</version>
</dependency>
```
Gradle:
```
compile 'com.ctrip.framework.apollo:apollo-client:${apollo.version}'
```
其中`${apollo.version}`是Apollo的版本号。
2. 实现ApolloConfigChangeListener接口
```java
import com.ctrip.framework.apollo.model.ConfigChangeEvent;
import com.ctrip.framework.apollo.spring.annotation.ApolloConfigChangeListener;
import org.springframework.stereotype.Component;
@Component
public class MyApolloConfigChangeListener {
@ApolloConfigChangeListener
private void onChange(ConfigChangeEvent changeEvent) {
// 配置变化时的回调方法
}
}
```
3. 实现BeanPostProcessor接口
```java
import org.springframework.beans.BeansException;
import org.springframework.beans.factory.config.BeanPostProcessor;
import org.springframework.beans.factory.config.ConfigurableListableBeanFactory;
import org.springframework.stereotype.Component;
@Component
public class MyBeanPostProcessor implements BeanPostProcessor {
private final ConfigurableListableBeanFactory beanFactory;
public MyBeanPostProcessor(ConfigurableListableBeanFactory beanFactory) {
this.beanFactory = beanFactory;
}
@Override
public Object postProcessBeforeInitialization(Object bean, String beanName) throws BeansException {
return bean;
}
@Override
public Object postProcessAfterInitialization(Object bean, String beanName) throws BeansException {
if (bean instanceof MyConfigurableBean) {
MyConfigurableBean myConfigurableBean = (MyConfigurableBean) bean;
String configValue = myConfigurableBean.getConfigValue(); // 从Apollo配置中心获取配置
myConfigurableBean.setConfigValue(configValue);
}
return bean;
}
@ApolloConfigChangeListener
private void onChange(ConfigChangeEvent changeEvent) {
if (changeEvent.isChanged("my.config.key")) {
beanFactory.destroySingleton("myConfigurableBean"); // 销毁旧的Bean实例
}
}
}
```
在这个例子中,我们实现了一个`MyConfigurableBean`,这个Bean的属性`configValue`是从Apollo配置中心获取的。当Apollo配置中心的配置发生变化时,我们会销毁旧的Bean实例,并重新创建新的Bean实例。
4. 配置Apollo
在`application.properties`或`application.yml`中配置Apollo相关参数:
```yaml
apollo:
meta:
# Apollo Portal的地址
http://localhost:8080
# Apollo AppId
appId: myAppId
# Apollo配置中心的命名空间
namespace: application
```
至此,我们就成功地接入了Apollo,实现了Spring依赖注入的Bean动态刷新。
阅读全文
相关推荐
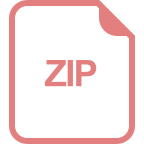
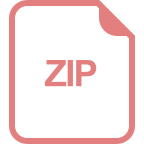

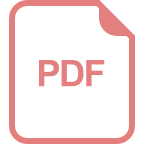
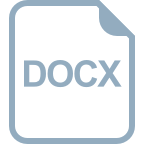
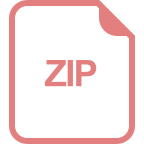
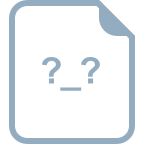
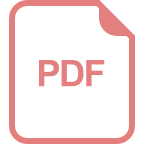
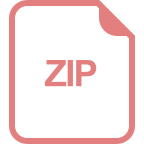
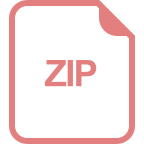
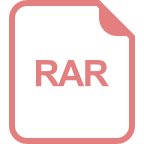
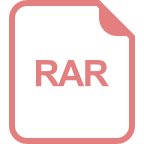
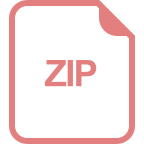
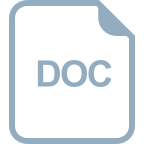
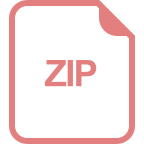